Structured programming
Introduction
Structured programming is a programming paradigm aimed at improving the clarity, quality, and development time of a computer program by making extensive use of subroutines, block structures, for and while loops, and other control structures. This paradigm was developed in the late 1960s and early 1970s as a means to address the complexities and inefficiencies associated with the unstructured programming practices of the time.
Historical Context
Structured programming emerged as a response to the difficulties encountered in the development and maintenance of large-scale software systems. Prior to its advent, programs were often written in an unstructured manner, leading to what was commonly referred to as "spaghetti code"—a tangled web of jumps and branches that made programs difficult to understand and modify.
The publication of Edsger W. Dijkstra's seminal paper "Go To Statement Considered Harmful" in 1968 marked a turning point in the adoption of structured programming principles. Dijkstra argued that the use of the GOTO statement should be minimized or eliminated in favor of more structured control flow constructs, such as loops and conditionals.
Core Principles
Structured programming is founded on several key principles that distinguish it from unstructured programming:
Sequence
The sequence control structure is the most basic building block of structured programming. It dictates that instructions are executed in a linear order, one after the other. This straightforward approach simplifies the flow of control and enhances readability.
Selection
Selection, or conditional branching, allows a program to choose between different paths of execution based on the evaluation of a condition. The most common selection constructs are the if-then-else statement and the switch-case statement. These constructs enable the program to make decisions and execute specific blocks of code accordingly.
Iteration
Iteration, or looping, enables a program to repeat a block of code multiple times. The primary iteration constructs in structured programming are the for loop, while loop, and do-while loop. These constructs allow for repetitive execution until a specified condition is met, facilitating tasks such as traversing arrays or processing data sets.
Structured Programming Languages
Several programming languages were designed with structured programming principles in mind. These languages provide built-in support for the core constructs of sequence, selection, and iteration, making it easier for developers to write structured code.
ALGOL
ALGOL (Algorithmic Language) is one of the earliest languages to embody structured programming principles. Developed in the late 1950s and early 1960s, ALGOL introduced block structures and nested control constructs, laying the groundwork for future structured programming languages.
Pascal
Pascal, designed by Niklaus Wirth in the late 1960s, was explicitly created to encourage structured programming practices. Pascal's syntax and features, such as strong typing and extensive use of subroutines, made it an ideal language for teaching structured programming concepts.
C
C is another language that supports structured programming. Developed in the early 1970s by Dennis Ritchie, C provides a rich set of control structures, including loops, conditionals, and functions, enabling developers to write clear and maintainable code.
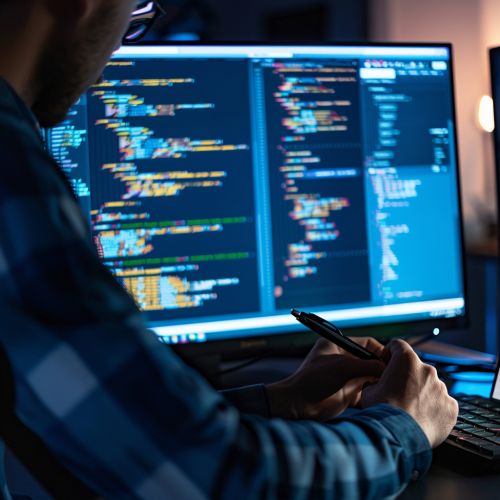
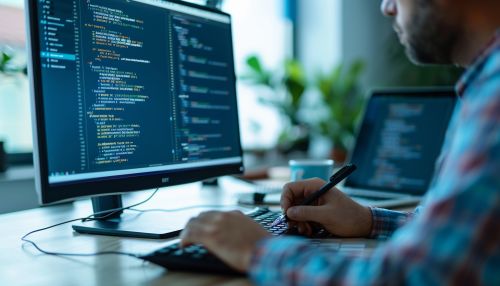
Benefits of Structured Programming
Structured programming offers several advantages over unstructured programming, contributing to its widespread adoption and enduring relevance.
Improved Readability
By organizing code into logical blocks and using clear control structures, structured programming enhances the readability of programs. This makes it easier for developers to understand and modify code, reducing the likelihood of errors and improving maintainability.
Enhanced Debugging and Testing
Structured programs are generally easier to debug and test due to their modular nature. Each subroutine or function can be tested independently, allowing for more efficient identification and resolution of issues. This modularity also facilitates the use of automated testing tools and techniques.
Reduced Complexity
The use of structured control constructs helps to reduce the overall complexity of a program. By avoiding the chaotic flow of control associated with unstructured programming, structured programs are more predictable and easier to reason about.
Reusability
Structured programming promotes the development of reusable code components. Functions and subroutines can be designed to perform specific tasks and then reused across different parts of a program or even in different projects. This reusability leads to more efficient development and reduces redundancy.
Criticisms and Limitations
While structured programming has many benefits, it is not without its criticisms and limitations. Some of the common critiques include:
Performance Overhead
The use of structured programming constructs can sometimes introduce performance overhead, particularly in resource-constrained environments. For example, the use of function calls and nested loops can lead to increased memory usage and slower execution times compared to highly optimized unstructured code.
Limited Expressiveness
Some critics argue that structured programming can be overly restrictive, limiting the expressiveness of a program. In certain cases, the rigid adherence to structured constructs may result in convoluted code that is less efficient or harder to understand than an equivalent unstructured solution.
Evolution of Programming Paradigms
The rise of other programming paradigms, such as object-oriented programming and functional programming, has led some to question the continued relevance of structured programming. These paradigms offer alternative approaches to organizing and managing code, each with its own set of advantages and trade-offs.
Structured Programming in Modern Software Development
Despite the emergence of new paradigms, structured programming remains a foundational concept in modern software development. Many contemporary programming languages and development practices continue to incorporate structured programming principles.
Integration with Object-Oriented Programming
Object-oriented programming (OOP) builds upon the principles of structured programming by introducing the concept of objects—self-contained units of code that encapsulate both data and behavior. Languages such as Java and C++ combine structured programming constructs with object-oriented features, allowing developers to leverage the benefits of both paradigms.
Influence on Coding Standards
Structured programming has had a lasting impact on coding standards and best practices. Many organizations and development teams adopt coding guidelines that emphasize the use of structured constructs, modular design, and clear, readable code. These standards help ensure consistency and maintainability across large codebases.
Role in Education
Structured programming continues to play a crucial role in computer science education. Introductory programming courses often focus on teaching structured programming concepts, providing students with a solid foundation before moving on to more advanced paradigms. Languages such as Python and JavaScript are commonly used in educational settings to illustrate structured programming principles.
Conclusion
Structured programming has had a profound and lasting impact on the field of software development. By promoting clarity, readability, and maintainability, it has helped to address many of the challenges associated with unstructured programming. While new paradigms have emerged, the principles of structured programming continue to influence modern programming languages, development practices, and educational curricula.