For loop
Overview
A for loop is a control flow statement used in many programming languages that allows code to be executed repeatedly. The term "for loop" comes from the English word "for", which is used as a keyword in most programming languages to introduce a for loop statement. The for loop is distinguished by an explicit loop counter or loop variable, allowing the body of the loop to know about the sequencing of each iteration.

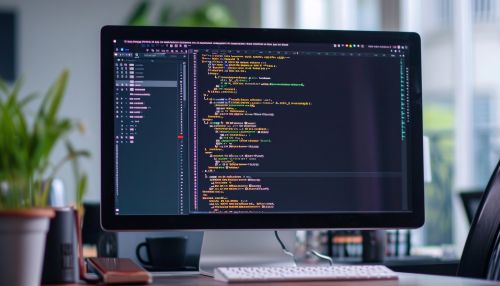
Syntax and semantics
The syntax of a for loop in most imperative programming languages involves three main parts: initialization, condition, and iteration expression. These three parts are combined in a single line of code such as the following:
```for (initialization; condition; iteration expression)```
The initialization is executed once at the beginning of the loop. The condition is checked before each iteration of the loop. If the condition is true, the loop statements are executed, and if it is false, the loop ends. The iteration expression is executed at the end of every loop iteration.
For loop in different programming languages
For loops are used in many programming languages, including C, C++, Java, Python, and JavaScript. The syntax and semantics of for loops can vary slightly between these languages.
C and C++
In C and C++, the syntax of a for loop is as follows:
```c for (initialization; condition; iteration expression) {
// loop statements
} ```
Java
In Java, the syntax of a for loop is similar to that in C and C++:
```java for (initialization; condition; iteration expression) {
// loop statements
} ```
Python
Python uses a different syntax for its for loop, which is more similar to a foreach loop in other languages:
```python for variable in sequence:
# loop statements
```
JavaScript
In JavaScript, the syntax of a for loop is similar to that in C, C++, and Java:
```javascript for (initialization; condition; iteration expression) {
// loop statements
} ```
Use cases
For loops are used in programming when a certain block of code needs to be executed a specified number of times. They are often used for iterating over arrays, performing calculations a certain number of times, or simply repeating an action.
Advantages and disadvantages
For loops have several advantages and disadvantages compared to other types of loops such as while loops and do-while loops.
Advantages
- For loops provide a concise way of writing the loop structure. Instead of always having to create and update a loop counter manually, you can define the start and end conditions of a loop in one line of code.
- The loop variable can be used within the loop to access the current iteration number.
Disadvantages
- For loops can be more complex than while loops and do-while loops, as they require three separate expressions to be maintained.
- If the loop variable is not used within the loop, a for loop may be less efficient than a while loop or a do-while loop, as the loop variable is always incremented or decremented.