C++
Overview
C++ is a general-purpose programming language that was developed by Bjarne Stroustrup at Bell Labs during the early 1980s. It is an extension of the C language, with enhancements such as classes and objects, which support the Object-Oriented paradigm. C++ is known for its efficiency and flexibility, allowing low-level and high-level programming.

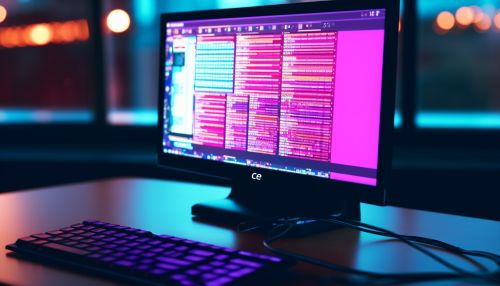
History
The development of C++ began in 1979 when Bjarne Stroustrup was working on his PhD thesis. Stroustrup found that the Simula language, which was the first language to support the concept of classes, was too slow for practical use. On the other hand, he found that the C language was efficient but lacked features for large-scale software development. As a result, he decided to create a new language that combined the efficiency of C with the features of Simula.
The first version of C++ was called "C with Classes". It was renamed to C++ in 1983, with the "++" indicating the increment operator in C, symbolizing the enhancements made to the C language.


Features
C++ includes several features that make it a powerful and flexible language. These include:
- Object-Oriented Programming: C++ supports the concept of classes and objects, which allows for data encapsulation, inheritance, and polymorphism.
- Efficiency: C++ allows for low-level programming, which can result in highly efficient code.
- Flexibility: C++ is a multi-paradigm language, supporting procedural, object-oriented, and generic programming.
- Portability: C++ code can be written to be platform-independent, allowing it to run on different operating systems and hardware.
- Standard Template Library (STL): The STL is a powerful feature of C++ that provides pre-defined classes and functions for common data structures and algorithms.


Syntax
The syntax of C++ is largely inherited from the C language, with additions for object-oriented and generic programming. The basic syntax includes:
- Variables: Variables in C++ must be declared before they can be used. The syntax for declaring a variable is: `type variable_name;`
- Functions: Functions in C++ are blocks of code that perform specific tasks. The syntax for declaring a function is: `return_type function_name(parameters) { // code }`
- Classes: Classes in C++ are user-defined types that encapsulate data and functions that operate on that data. The syntax for declaring a class is: `class ClassName { // data and functions };`
- Templates: Templates in C++ allow functions and classes to operate on generic types. The syntax for declaring a template is: `template <typename T> // function or class`


Applications
C++ is used in a wide range of applications, including:
- System Software: Operating systems, file systems, and network drivers are often written in C++ due to its efficiency and control over system resources.
- Game Development: C++ is a popular language for game development, due to its speed and the control it offers over hardware resources.
- Web Browsers: The rendering engines of many web browsers are written in C++, including those of Chrome and Firefox.
- Databases: Many high-performance databases, such as MySQL, are written in C++.
- Machine Learning: Libraries for machine learning, such as TensorFlow, are written in C++ for performance reasons.

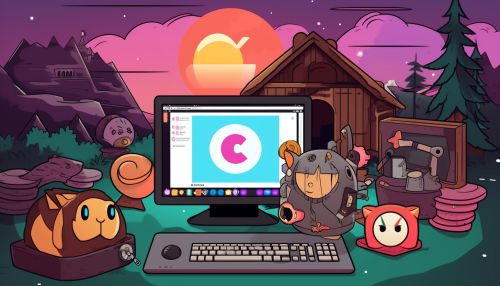