Standard Template Library
Overview
The Standard Template Library (STL) is a software library that is part of the C++ Standard Library. It provides several generic classes and functions, which allows programmers to manipulate data structures and perform algorithmic tasks on them in a more efficient and easier way. The STL achieves its goals through the heavy use of C++ templates, which makes it possible to write generic and reusable code.
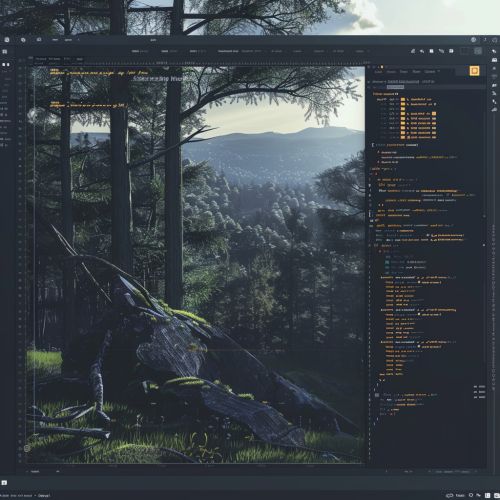
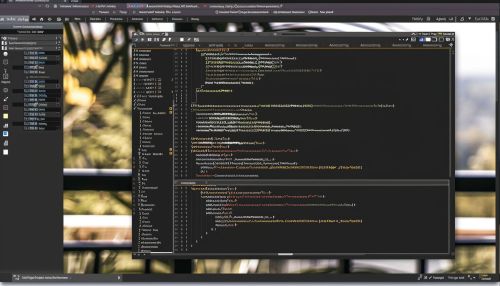
History
The STL was created by Alexander Stepanov, Meng Lee, and David Musser at Hewlett-Packard Laboratories in the 1990s. It was then proposed to the ISO C++ standards committee and was included in the C++ Standard Library in 1998. The STL has been a part of the C++ Standard Library since the standardization of C++ in 1998.
Components
The STL consists of four major components: algorithms, containers, functions, and iterators.
Algorithms
Algorithms are template functions that provide common operations on sequences of elements. These operations include sorting, searching, modifying, and several others. The algorithms are designed to be generic and can work with any data type that meets the requirements of the algorithm.
Containers
Containers are data structures that store collections of objects. The STL provides several types of containers, including vectors, lists, queues, stacks, sets, maps, and others. Each container has its own characteristics and should be used in situations where its characteristics provide the most benefits.
Functions
Functions, also known as function objects or functors, are objects that can be called as if they were ordinary functions. In the STL, function objects are used for many purposes, including serving as arguments for algorithms that need to perform an operation on the elements of a container.
Iterators
Iterators are objects that can be used to access the elements of a container in a sequential manner. They are a key component of the STL and make it possible for the algorithms to work with the containers in a generic way.
Usage
The STL is used by including the appropriate header files in the C++ program and then using the STL components as needed. The STL is designed to be easy to use and to provide high performance. However, it also requires a good understanding of C++ templates and other advanced features of the language.
Advantages and Disadvantages
The STL has several advantages, including code reuse, efficiency, and flexibility. However, it also has some disadvantages, such as complexity and the potential for misuse.
Advantages
The main advantage of the STL is that it provides a set of generic algorithms and data structures that can be used with any data type. This allows for a high degree of code reuse, as the same algorithm can be used with different types of data. The STL also provides a high level of performance, as the algorithms and data structures are designed to be efficient.
Disadvantages
The main disadvantage of the STL is its complexity. The use of templates and other advanced features of C++ can make the STL difficult to understand and use correctly. In addition, the generic nature of the STL can lead to misuse if the requirements of the algorithms and data structures are not fully understood.