Data Structures
Introduction
Data structures are fundamental concepts in the field of computer science that allow efficient organization, storage, and retrieval of data. They are designed to facilitate the management of data in a way that enhances the efficiency of specific operations such as searching and sorting. Data structures provide a means of managing large amounts of data efficiently for uses such as large databases and internet indexing services.

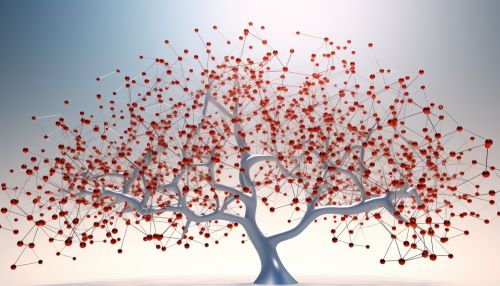
Types of Data Structures
There are two types of data structures: primitive and non-primitive data structures.
Primitive Data Structures
Primitive data structures are the simplest forms of data structures and are directly operated upon by machine-level instructions. They include:
- Integer: This is a numerical data type that represents some finite subset of the mathematical integers.
- Float: This is a data type composed of a number that is not an integer, because it includes a fraction represented in decimal format.
- Character: This is a data type that holds letters and symbols in a character set, including digits and punctuation.
- Boolean: This is a data type that has one of two possible values (usually denoted true and false), intended to represent the two truth values of logic and Boolean algebra.
Non-Primitive Data Structures
Non-primitive data structures are more complex types of data structures that are derived from primitive data structures. They can be divided into linear and non-linear data structures.
Linear Data Structures
Linear data structures are those in which data elements form a sequence or a linear list. Examples include:
- Arrays: An array is a collection of items stored at contiguous memory locations. The idea is to store multiple items of the same type together. This makes it easier to calculate the position of each element by simply adding an offset to a base value.
- Linked Lists: A linked list is a linear data structure, in which the elements are not stored at contiguous memory locations. The elements in a linked list are linked using pointers.
- Stacks: A stack is a basic data structure that can be logically thought of as a linear structure represented by a real physical stack or pile, a structure where insertion and deletion of items takes place at one end called the top of the stack.
- Queues: A queue is a common type of data structure that places elements in a sequence, similar to a stack. While a stack is a LIFO (Last in First Out) structure, a queue is a FIFO (First in First Out) structure.

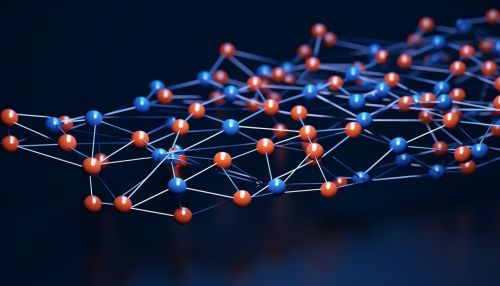
Non-Linear Data Structures
Non-linear data structures are those in which data elements do not form a sequence. Examples include:
- Trees: A tree is a widely used abstract data type (ADT) that simulates a hierarchical tree structure, with a set of linked nodes. This is a "non-linear" data structure compared to arrays, linked lists, stacks and queues which are linear data structures.
- Graphs: In graph theory, a graph is a series of vertexes connected by edges. Graphs can be either directed or undirected.
Applications of Data Structures
Data structures are used in almost every program or software system that has been written. They are critical to the design and implementation of efficient software applications. Some of the more common uses of data structures include:
- Compiler Design: Many compiler design problems can be solved using various types of data structures. For example, symbol tables of compilers are implemented using a hash table.
- Operating System: The operating system uses data structures like queues, buffers, stacks, and hash tables for task scheduling, memory management, and lots of other stuff.
- Database Management System: Data structures are used extensively in the Database Management System. For example, B-trees are used to index data.
- Network Data Model: The network data model uses a graph data structure to represent the relationship between records.
- Artificial Intelligence: AI uses data structures like decision trees, game trees, neural networks, etc.
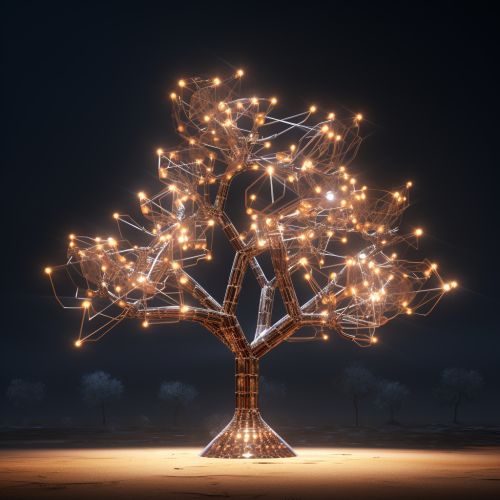

Efficiency of Data Structures
The efficiency of a data structure cannot be analyzed separately from those operations. This is why we measure the time complexity of these operations. The time complexity of an operation can be defined as the number of computer instructions executed per operation. The space complexity of a data structure is the amount of memory used by the data structure.
Conclusion
Data structures are a crucial part of programming and are used in almost every aspect of Computer Science. Understanding the different types of data structures and their uses can help programmers choose the most efficient data structure for their specific needs, leading to more efficient and effective programs.
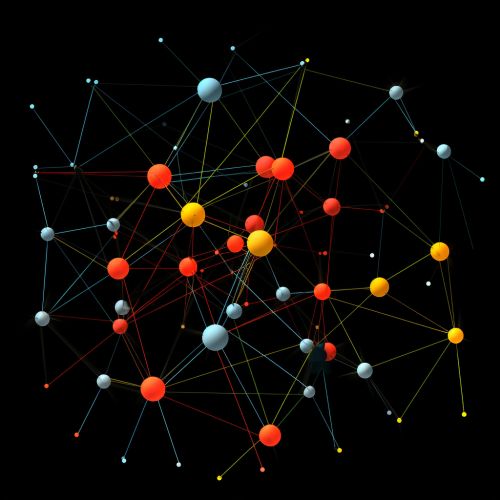
