Proxy Pattern
Definition
The Proxy Pattern is a design pattern in software engineering that provides a surrogate or placeholder for another object to control access to it. This pattern falls under the category of structural patterns because it deals with object composition, or how the entities can use each other.
Overview
The Proxy Pattern introduces a level of indirection when accessing an object. The indirection can be used to control the operations of the object, provide additional functionality, or delay the full cost of creation and initialization of the object until we actually need to use it.
Structure
The Proxy Pattern involves a series of components:
- Subject: This is an interface that both the RealObject and Proxy classes implement. It defines the common interface so that the Proxy can be used anywhere the RealObject is expected.
- RealObject: This is the real object that the proxy represents.
- Proxy: This is the class that holds a reference to the RealObject, and can control access to the RealObject. It can also provide additional functionality, and is responsible for creation and maintenance of the RealObject.

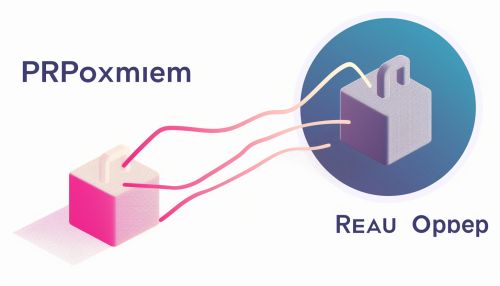
Types of Proxy
There are several types of proxies that can be implemented using the Proxy Pattern:
- Virtual Proxy: This type of proxy creates expensive objects on demand. The RealObject is only created when a client first requests/accesses the object.
- Remote Proxy: This provides a local representative for an object that resides in a different address space. This is what Java's Remote Method Invocation (RMI) uses.
- Protective Proxy: This type of proxy controls access to a sensitive master object. The 'surrogate' object checks that the caller has the access permissions required prior to forwarding the request.
- Smart Reference: This type of proxy provides additional actions whenever an object is accessed. Typical uses include counting the number of references to an object, locking the object to ensure that no other object can change it, and loading a persistent object into memory when it's first referenced.
Implementation
The implementation of the Proxy Pattern can be quite straightforward. In the Java programming language, for example, the Proxy class can be implemented as follows:
```java public interface Subject {
void request();
}
public class RealObject implements Subject {
public void request() { System.out.println("RealObject request"); }
}
public class Proxy implements Subject {
private RealObject realObject;
public void request() { if (realObject == null) { realObject = new RealObject(); } realObject.request(); }
} ```
In this example, the Proxy class controls access to the RealObject. The RealObject is only created when the request method is called on the Proxy class.
Use Cases
The Proxy Pattern is used when we need to manage and control access to an object. Some practical applications of the Proxy Pattern include:
- Lazy Initialization: The proxy controls the instantiation of expensive objects, creating them on demand.
- Access Control: The proxy controls access to the original object, allowing certain operations and denying others.
- Local Execution of Remote Services: The proxy represents information that's expensive to create.
- Logging: The proxy provides a way to add logging functionality when an object is accessed.
Advantages and Disadvantages
Like any design pattern, the Proxy Pattern has its pros and cons.
Advantages:
- It provides a level of security by protecting the real component from harmful misuse.
- It reduces the memory and storage footprint of an application by handling memory-intensive operations.
- It isolates the code that handles the physical storage and retrieval of data from the rest of the application, improving modularity and making the application easier to evolve and understand.
Disadvantages:
- It can introduce a level of complexity and overhead that may not be justified if the RealObject is not complex or does not require indirect access for some reason.
- It may be difficult to implement a proxy when the RealObject has complex interactions with other objects.