Java RMI
Introduction
Java Remote Method Invocation (RMI) is a Java API that performs the object-oriented equivalent of remote procedure calls (RPC). It allows an object residing in one Java Virtual Machine (JVM) to invoke methods on an object residing in another JVM. This capability is essential for developing distributed applications, where components can interact across network boundaries.
Architecture
Java RMI architecture is based on a layered model, with each layer having specific responsibilities. The layers include:
Stub and Skeleton Layer
The stub and skeleton layer is responsible for intercepting method calls made by the client and redirecting them to a remote RMI service. The stub acts as a proxy for the remote object, while the skeleton is responsible for dispatching the call to the actual remote object implementation.
Remote Reference Layer
The remote reference layer manages references to remote objects. It handles the creation, management, and communication of remote object references, ensuring that method calls are correctly routed to the appropriate remote object.
Transport Layer
The transport layer is responsible for setting up the connection between the client and server JVMs. It manages the underlying network communication, including opening and closing sockets, handling data transmission, and ensuring data integrity.
RMI Registry
The RMI registry is a simple server-side name service that allows clients to obtain a reference to a remote object. Remote objects are registered with the RMI registry under a unique name, which clients use to look up and obtain a reference to the remote object.
Remote Interfaces
In Java RMI, remote interfaces define the methods that can be invoked remotely. A remote interface must extend the java.rmi.Remote interface and declare that each method can throw a java.rmi.RemoteException. This ensures that network-related exceptions are properly handled.
Implementation Classes
Implementation classes provide the actual implementation of the remote methods defined in the remote interface. These classes must extend the java.rmi.server.UnicastRemoteObject class, which provides the necessary functionality for remote communication.
RMI Security
Java RMI includes several security features to protect against unauthorized access and ensure data integrity. These features include:
Security Manager
The security manager is responsible for enforcing security policies, such as restricting access to certain resources or preventing unauthorized method calls. Developers can customize the security manager to implement specific security requirements.
Codebase
The codebase feature allows clients to download the necessary classes for remote objects from a specified URL. This ensures that clients have the correct version of the classes needed to interact with remote objects.
RMI Activation
RMI activation allows remote objects to be activated on demand, rather than being always active. This can help conserve resources and improve performance. The activation framework includes:
Activatable Class
The java.rmi.activation.Activatable class provides the necessary functionality for creating activatable remote objects. These objects can be registered with an activation system, which manages their lifecycle and ensures they are activated when needed.
Activation System
The activation system is responsible for managing the activation and deactivation of remote objects. It includes an activation daemon, which listens for activation requests and activates the necessary objects.
Performance Considerations
Java RMI performance can be influenced by several factors, including network latency, serialization overhead, and the complexity of remote method calls. Developers can optimize performance by:
- Minimizing the amount of data transmitted over the network.
- Using efficient serialization mechanisms.
- Reducing the frequency of remote method calls.
Use Cases
Java RMI is used in various applications, including:
- Distributed computing: Enabling components to interact across network boundaries.
- Enterprise applications: Facilitating communication between different parts of an enterprise system.
- Middleware: Providing a foundation for other distributed technologies, such as Enterprise JavaBeans (EJB).
Alternatives
While Java RMI is a powerful tool for developing distributed applications, there are several alternatives, including:
- CORBA: A language-agnostic standard for distributed objects.
- SOAP: A protocol for exchanging structured information in web services.
- gRPC: A high-performance RPC framework developed by Google.
See Also
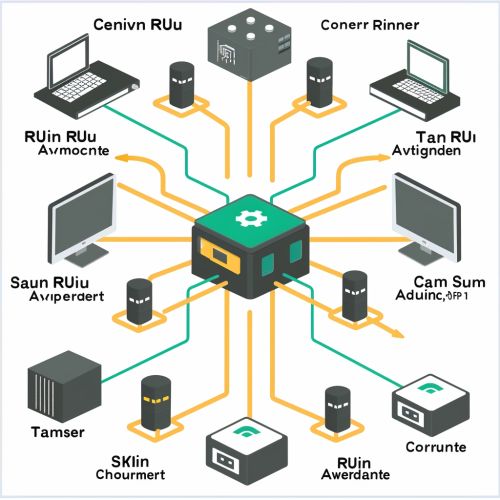
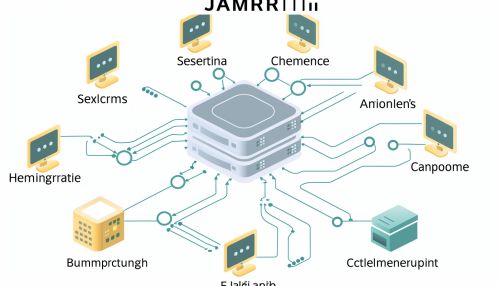