Facade Pattern
Introduction
The **Facade Pattern** is a structural design pattern commonly used in software engineering. It provides a simplified interface to a complex subsystem, making it easier to use and understand. This pattern is part of the Gang of Four design patterns and is widely utilized in object-oriented programming to promote loose coupling and enhance code readability.
Definition and Purpose
The primary purpose of the Facade Pattern is to provide a unified interface to a set of interfaces in a subsystem. By doing so, it hides the complexities of the subsystem from the client, making the subsystem easier to use. This pattern is particularly useful when a system is very complex or difficult to understand, as it abstracts the underlying implementation and exposes only the necessary functionalities.
Structure
The Facade Pattern typically involves three main components: 1. **Facade**: The class that provides a simplified interface to the complex subsystem. 2. **Subsystem Classes**: The classes that perform the actual work and contain the complex logic. 3. **Client**: The class that interacts with the Facade to access the functionalities of the subsystem.
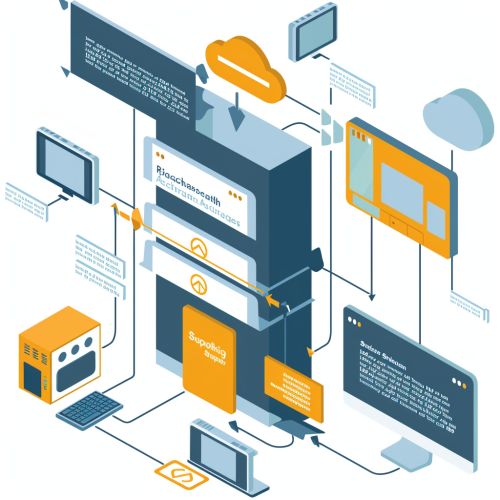
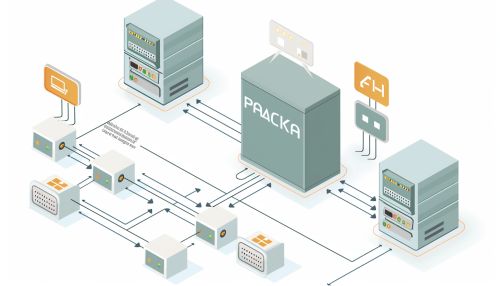
Implementation
To implement the Facade Pattern, follow these steps: 1. **Identify the Subsystem**: Determine the classes and methods that form the complex subsystem. 2. **Create the Facade Class**: Design a class that provides a simple interface to the subsystem. This class should delegate client requests to the appropriate subsystem classes. 3. **Refactor the Client Code**: Modify the client code to interact with the Facade instead of directly with the subsystem classes.
Here is an example in Java: ```java // Subsystem classes class SubsystemA {
public void operationA() { // Complex logic }
}
class SubsystemB {
public void operationB() { // Complex logic }
}
// Facade class class Facade {
private SubsystemA subsystemA; private SubsystemB subsystemB;
public Facade() { subsystemA = new SubsystemA(); subsystemB = new SubsystemB(); }
public void simpleOperation() { subsystemA.operationA(); subsystemB.operationB(); }
}
// Client code public class Client {
public static void main(String[] args) { Facade facade = new Facade(); facade.simpleOperation(); }
} ```
Benefits
The Facade Pattern offers several advantages: 1. **Simplifies Usage**: By providing a simplified interface, it makes the subsystem easier to use and understand. 2. **Reduces Coupling**: It decouples the client from the complex subsystem, promoting loose coupling. 3. **Improves Maintainability**: Changes to the subsystem can be made without affecting the client code, as long as the Facade interface remains consistent. 4. **Enhances Readability**: It improves code readability by hiding the complex implementation details from the client.
Drawbacks
While the Facade Pattern has many benefits, it also has some drawbacks: 1. **Over-Simplification**: It might oversimplify the subsystem, limiting the client's ability to use advanced features. 2. **Performance Overhead**: The additional layer of abstraction can introduce a slight performance overhead. 3. **Increased Complexity**: In some cases, introducing a Facade can add an extra layer of complexity to the system.
Real-World Examples
The Facade Pattern is widely used in various real-world applications: 1. **Graphical User Interfaces (GUIs)**: In GUI frameworks, a Facade can simplify the interaction with complex subsystems like event handling and rendering. 2. **Database Access**: A Facade can provide a simplified interface for database operations, hiding the complexities of connection management and query execution. 3. **Networking**: In networking libraries, a Facade can abstract the complexities of socket programming and protocol handling.
Related Patterns
The Facade Pattern is often used in conjunction with other design patterns: 1. **Adapter Pattern**: While the Facade Pattern provides a simplified interface, the Adapter Pattern converts one interface into another that the client expects. 2. **Decorator Pattern**: The Decorator Pattern can be used to add responsibilities to objects dynamically, complementing the Facade by enhancing its functionalities. 3. **Singleton Pattern**: The Singleton Pattern ensures that a class has only one instance, which can be useful for Facade classes to provide a single point of access to the subsystem.