Programming Paradigm
Overview
A programming paradigm is a fundamental style of computer programming, which is not generally dictated by the project management methodology, structure, or domain of the project. Paradigms differ in the concepts and abstractions used to represent the elements of a program (such as objects, functions, variables, constraints, etc.) and the steps that compose a computation (such as assignations, evaluations, continuations, data flows, etc.). Some paradigms are concerned mainly with implications for the execution model of the language, such as allowing side effects, or whether the sequence of operations is defined by the execution model. Other paradigms are concerned mainly with the way that code is organized, such as grouping a code into units along with the state that is modified by the code. Yet others are concerned mainly with the style of syntax and grammar.
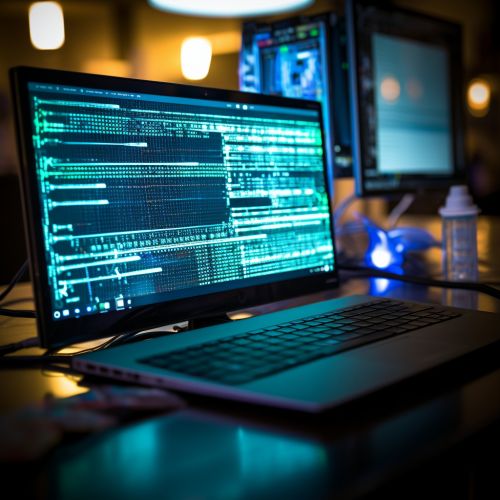

History
The first programming languages were assembly languages, not far removed from instructions directly executed by hardware. The very first high-level programming language was Fortran. Developed in the 1950s, it aimed to allow programmers to use a language that was more akin to English, with terms like 'DO WHILE' replacing more obtuse machine code. As new programming languages have developed, more paradigms have emerged, some of which are detailed below.
Imperative Programming
Imperative programming is a programming paradigm that uses statements that change a program's state. In much the same way that the imperative mood in natural languages expresses commands, an imperative program consists of commands for the computer to perform. Imperative programming focuses on describing how a program operates. It is a procedural programming paradigm, characterized by a linear top-down approach and global state changes, which distinguishes it from functional programming. The most common examples of imperative programming languages are C, Java, and Python.
Object-Oriented Programming
Object-oriented programming (OOP) is a programming paradigm based on the concept of "objects", which can contain data and code: data in the form of fields (often known as attributes or properties), and code, in the form of procedures (often known as methods). A distinguishing feature of objects is that an object's procedures can access and often modify the data fields of the object with which they are associated. Thus, object-oriented computer programs are made out of objects that interact with each other. OOP languages are diverse, but the most popular ones are class-based, meaning that objects are instances of classes, which also determine their types. Some of the most popular OOP languages include Java, C++, and Python.
Functional Programming
Functional programming is a programming paradigm where programs are constructed by applying and composing functions. It is a declarative programming paradigm in which function definitions are trees of expressions that each return a value, rather than a sequence of imperative statements which change the state of the program. In functional programming, functions are first-class citizens, meaning that they can be bound to names (including local identifiers), passed as arguments, and returned from other functions, just as any other data type can. This is in contrast to imperative programming, where programs are composed of statements which change global state when executed. Functional programming is a subset of declarative programming. Examples of functional languages include Haskell, Lisp, and Scala.
Logic Programming
Logic programming is a programming paradigm which is largely based on formal logic. Any program written in a logic programming language is a set of sentences in logical form, expressing facts and rules about some problem domain. Major logic programming language families include Prolog, Answer set programming (ASP) and Datalog. In all of these languages, rules are written in the form of clauses:
H :- B1, ..., Bn.
and are read declaratively as logical implications.
Concurrent Programming
Concurrent programming is a form of computing in which several computations are executed concurrently—during overlapping time periods—instead of sequentially, with one completing before the next starts. This is a property of systems—such as process, operating system, and application—that is separate from the number of processors or cores available in the hardware, which are used for parallel computing. Most current programming languages have some form of concurrency, such as Java's threads.