Object-Oriented Programming
Introduction
Object-oriented programming (OOP) is a programming paradigm based on the concept of "objects", which can contain data and code: data in the form of fields (often known as attributes or properties), and code, in the form of procedures (often known as methods). This approach aims to implement real-world entities like inheritance, hiding, polymorphism etc in programming. It is fundamentally different from procedural programming where, instead of dividing the program into procedures, it is divided into objects.
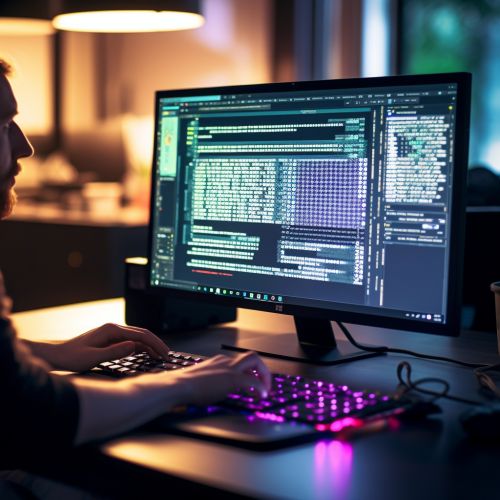
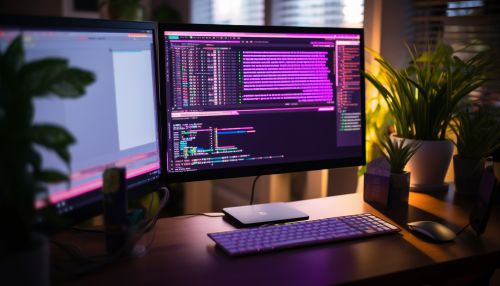
History
The concept of object-oriented programming was introduced in the late 1960s with the advent of the Simula language. Simula was the first language to introduce the concepts of classes and objects. However, it was the Smalltalk language, developed at Xerox PARC, that popularized object-oriented programming and brought it into the mainstream.
Principles of Object-Oriented Programming
Object-oriented programming is based on four major principles:
Encapsulation
Encapsulation is the mechanism of hiding data implementation by restricting access to public methods. Instance variables are kept private and accessor methods are made public to achieve this.
Inheritance
Inheritance is a mechanism where one class acquires the property of another class. With the use of inheritance, information is made manageable in a hierarchical order.
Polymorphism
Polymorphism allows methods to be used in the same way even though they may be performing different tasks. It reduces complexities by allowing the same method to behave differently, based on the object that is calling it.
Abstraction
Abstraction is a mechanism that represents essential features without including background details. It is a process of hiding the implementation details and showing only the functionality to the user.
Concepts of Object-Oriented Programming
Object-oriented programming is built around a number of concepts, including objects, classes, inheritance, encapsulation, abstraction, and polymorphism.
Objects
In object-oriented programming, an object is an instance of a class. Objects are an encapsulation of variables and functions into a single entity. Objects get their variables and functions from classes.
Classes
A class is a blueprint for creating objects. It defines a set of properties and methods that are common to all objects of one type.
Inheritance
Inheritance is a way to form new classes using classes that have already been defined. The new classes, known as derived classes, inherit attributes and behavior of the pre-existing classes, which are referred to as base classes.
Encapsulation
Encapsulation is an object-oriented programming concept that binds together the data and functions that manipulate the data, and that keeps both safe from outside interference and misuse.
Abstraction
Abstraction is a process of hiding the implementation details from the user, only the functionality will be provided to the user.
Polymorphism
Polymorphism is a concept by which we can perform a single action in different ways. It provides a way to structure code so it is more readable and maintainable.
Advantages of Object-Oriented Programming
Object-oriented programming has several advantages over procedural programming:
- OOP is faster and easier to execute - OOP provides a clear structure for the programs - OOP helps to keep the code DRY (Don't Repeat Yourself), and makes the code easier to maintain, modify and debug - OOP makes it possible to create full reusable applications with less code and shorter development time
Disadvantages of Object-Oriented Programming
Despite the many advantages, object-oriented programming also has a few disadvantages:
- OOP can be considered more complex to learn and understand than procedural programming, especially for beginners - OOP requires more memory and processing power than procedural programming - OOP can be overkill for very simple, non-graphical programs
Conclusion
Object-oriented programming is a powerful and flexible paradigm that provides a way to structure software so that properties and behaviors are bundled into individual objects. It offers several benefits over procedural programming, including code reusability, modularity, and efficiency. However, it also has its disadvantages and may not be suitable for all types of software development.
See Also
- Programming paradigm - Procedural programming - Simula - Smalltalk - Encapsulation (computer programming) - Inheritance (object-oriented programming) - Polymorphism (computer science) - Abstraction (computer science)