Functional Programming
Introduction
Functional programming is a programming paradigm that treats computation as the evaluation of mathematical functions and avoids changing-state and mutable data. It is a declarative type of programming paradigm that emphasizes functions that produce results that depend only on their inputs and not on the program state - i.e., pure mathematical functions.
Concepts
Functional programming involves several key concepts that distinguish it from other programming paradigms:
First-Class and Higher-Order Functions
In functional programming, functions are first-class citizens. This means that, like any other value, they can be passed as arguments to other functions, returned as values from other functions, and assigned to variables. Higher-order functions are a direct result of this characteristic. A higher-order function is a function that takes one or more functions as arguments, returns a function as its result, or both.
Pure Functions
Pure functions are a key part of functional programming. A function is considered pure if its return value is solely determined by its input values, and it does not produce any side effects. In other words, given the same input, a pure function will always return the same output.
Immutability
In functional programming, once a variable is assigned a value, it cannot be changed. This is known as immutability. This characteristic avoids any changes in state, which can introduce bugs and complexities in the code.
Recursion
Since functional programming discourages the use of loops that change state, recursion is often used as a substitute. Recursion is the process of a function calling itself as a subroutine. This can be used to solve complex problems by breaking them down into simpler, smaller problems.
Referential Transparency
Referential transparency is a property of pure functions in functional programming. A function call is referentially transparent if it can be replaced with its corresponding value without changing the program's behavior.
Functional Programming Languages
There are several programming languages that are designed to support the functional programming paradigm:
Lisp
Lisp is one of the oldest programming languages still in use today. It was created in 1958 by John McCarthy at MIT. Lisp is known for its simple syntax, where every expression is written as a function call.
Haskell
Haskell is a statically-typed functional programming language that was released in 1990. It is known for its strong support for pure functions, immutability, and lazy evaluation.
Erlang
Erlang is a functional programming language that was designed for building robust, fault-tolerant systems. It was originally developed by Ericsson in the 1980s to handle telecommunication switches, but it is now used in various domains, including e-commerce, banking, and instant messaging.
Scala
Scala is a modern programming language that combines functional programming and object-oriented programming. It runs on the Java Virtual Machine (JVM) and can interoperate with Java code.
Advantages and Disadvantages
Like any programming paradigm, functional programming has its advantages and disadvantages.
Advantages
Functional programming offers several benefits:
- It simplifies the debugging process because pure functions do not change the state of the program. - It makes the code more readable and easier to understand. - It can make the code more efficient and easier to optimize. - It can make the code more testable because pure functions are easier to reason about.
Disadvantages
However, functional programming also has some drawbacks:
- It can be difficult to learn, especially for programmers who are used to imperative programming paradigms. - It can lead to performance issues, especially with recursion and memory usage. - It can be more difficult to handle input/output operations, which are inherently stateful.
See Also
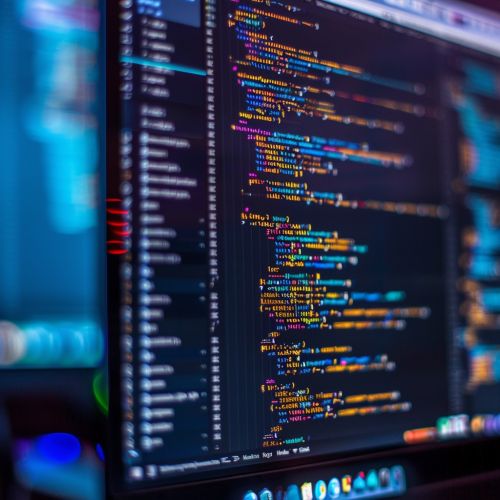