List (abstract data type)
Definition
A list is an abstract data type that represents a countable number of ordered values, where the same value may occur more than once. An instance of a list is a computer representation of the mathematical concept of a finite sequence; the (potentially) infinite analog of a list is a stream.
Characteristics
Each element in a list typically has a link or a reference to the next element in the sequence, this property gives rise to the name 'linked list'. The operations available on a list data structure can include adding or removing elements at any position in the sequence, or iterating over the elements in various orders.
Types of Lists
There are several types of lists which include but are not limited to:
Array Lists
An array list is a type of list that is implemented using an array as the underlying data structure. The advantage of an array list is that it provides constant time access to any element in the list by its index. However, the disadvantage is that adding or removing elements from the list can be a costly operation as it may require shifting elements to maintain the list's order.
Linked Lists
A linked list is a type of list where each element is a separate object called a node. Each node contains a reference to the next node in the list. The advantage of a linked list is that adding or removing elements is a constant time operation. However, the disadvantage is that accessing elements by their index is a linear time operation.
Doubly Linked Lists
A doubly linked list is a type of list where each node has a reference to both the next node and the previous node in the list. This allows for more efficient traversal in both directions but at the cost of increased complexity and memory usage.
Circular Lists
A circular list is a type of list where the last element in the list has a reference to the first element, effectively creating a loop. This can be useful in certain algorithms where a continuous loop is needed.
Operations
The following operations are typically available on lists:
Access
Accessing an element in a list typically involves specifying the index of the element. The time complexity of this operation can vary depending on the type of list. For example, in an array list, access is a constant time operation, while in a linked list, it is a linear time operation.
Insertion
Inserting an element into a list involves adding the element at a specific position in the list. The time complexity of this operation can also vary. In an array list, insertion can be a costly operation as it may require shifting elements, while in a linked list, it is a constant time operation.
Deletion
Deleting an element from a list involves removing the element at a specific position. Like insertion, the time complexity of this operation can vary. In an array list, deletion can be a costly operation due to the potential need to shift elements, while in a linked list, it is a constant time operation.
Search
Searching for an element in a list involves checking each element in the list until the desired element is found. The time complexity of this operation is typically linear as it requires checking each element in the list.
Applications
Lists are used in various applications in computer science. They are used to implement other data structures like stacks and queues. They are also used in various algorithms for tasks like sorting and searching.
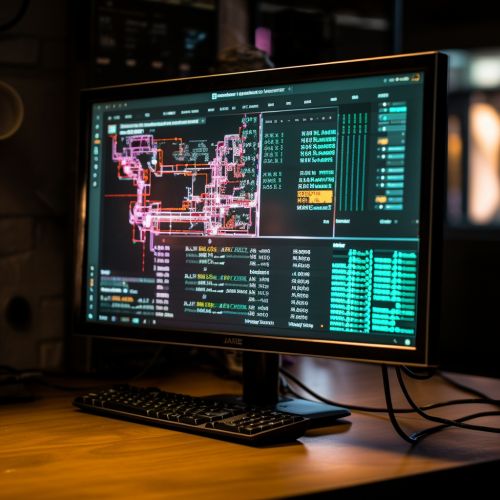
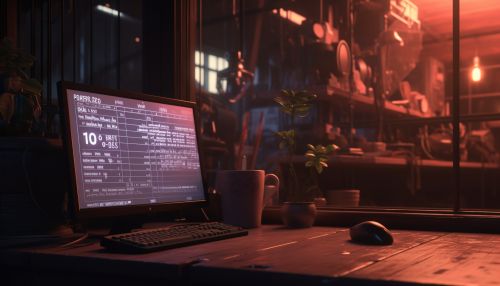