Queue (abstract data type)
Overview
A queue is an abstract data type that serves as a collection of elements, with two principal operations: enqueue, which adds an element to the collection, and dequeue, which removes the earliest added element. The term queue takes its name from the real-world analogy, as line of people waiting for a service, where the first person to arrive is the first one to be served.
Characteristics
A queue is characterized by its FIFO (First In, First Out) property. This means that the order in which elements are added to the queue is the order in which they are removed. This is in contrast to a stack, another abstract data type, which operates on a LIFO (Last In, First Out) basis.
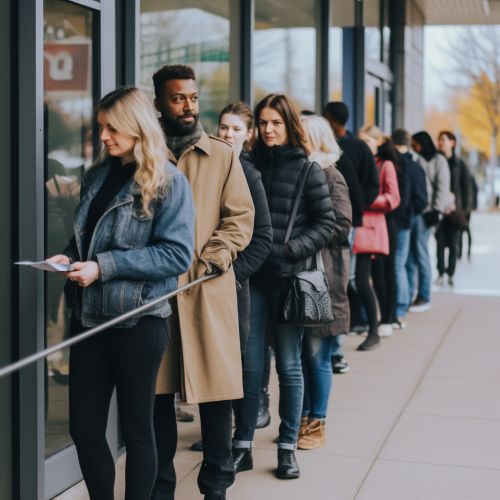
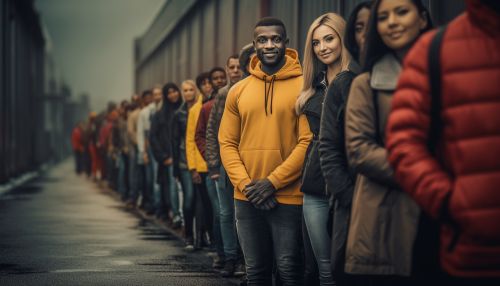
Operations
The two primary operations associated with a queue are:
- Enqueue: This operation adds an element to the end of the queue.
- Dequeue: This operation removes an element from the front of the queue.
In addition to these, there are several other operations that can be performed on a queue, such as:
- Peek / Front / Top: This operation returns the value of the front element without dequeuing it.
- IsEmpty: This operation checks if the queue is empty.
- IsFull: This operation checks if the queue is full.
Types of Queues
There are several types of queues, each with its own specific use case:
- Simple Queue: This is the basic type of queue where the insertion takes place at the rear and removal occurs at the front.
- Circular Queue: In a circular queue, the last element points to the first element making a circular link.
- Priority Queue: In a priority queue, elements are dequeued based on their priority rather than their order in the queue.
- Double Ended Queue (Deque): In a deque, insertion and removal of elements can be performed from either from the front or rear.
Applications
Queues are widely used in computer science and are pivotal for processes such as scheduling tasks, handling asynchronous data transfers, and serving as buffers for I/O operations. They are also used in non-computer science applications such as serving requests in a web server or handling calls in a call center.
Implementation
Queues can be implemented using different data structures like arrays, linked-lists, or stacks. The choice of implementation will depend on the specific requirements of the algorithm or application.