Array
Definition
An Array is a fundamental data structure, which can store a fixed-size sequential collection of elements of the same type. An array is used to store a collection of data, but it is often more useful to think of an array as a collection of variables of the same type.
Structure
All arrays consist of contiguous memory locations. The lowest address corresponds to the first element and the highest address to the last element. Arrays can be declared in various ways in different languages. For example, in C, the syntax for declaring an array is:
```c type arrayName [ arraySize ]; ```
This is called a single-dimensional array. The arraySize must be an integer constant greater than zero and type can be any valid C data type. For example, to declare a 10-element array called balance of type double, use this statement:
```c double balance[10]; ```
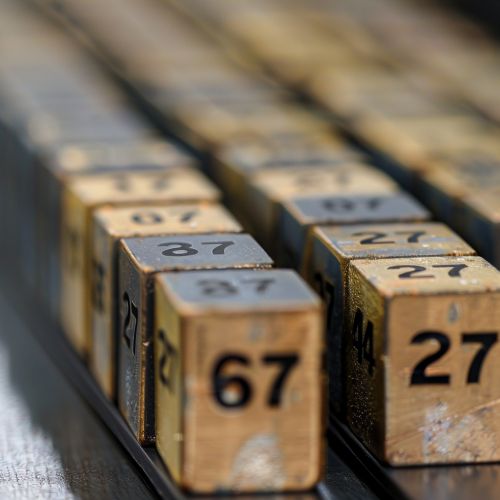
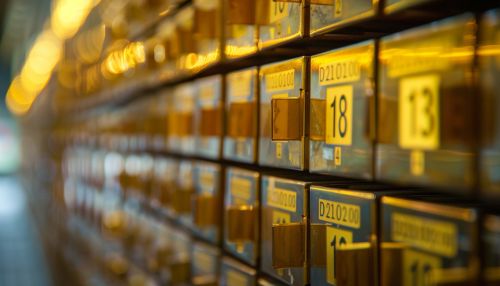
Accessing Array Elements
An element is accessed by indexing the array name. This is done by placing the index of the desired element within square brackets after the name of the array. For example:
```c double salary = balance[9]; ```
The above statement will take the 10th element from the array and assign the value to salary variable.
Arrays in Different Programming Languages
Different programming languages handle arrays in a variety of ways. Some languages, like Python and JavaScript, allow for mixed-type arrays, meaning that an array can hold elements of different data types. Other languages, like Java and C, require all elements of an array to be of the same type.
C
In C, arrays are a lower-level data structure and do not have built-in functions. The programmer must manage the array's size and handle its memory allocation.
Python
Python, on the other hand, provides a higher-level data structure called a list that can hold any type of object. Python lists are mutable and have a variety of useful methods, such as append(), extend(), and remove().
JavaScript
JavaScript arrays are a global object and can hold any type of data. They are dynamically sized and have a variety of built-in methods for manipulation, such as push(), pop(), shift(), unshift(), and splice().
Java
Java arrays are objects that store multiple variables of the same type. However, an array itself is an object on the heap.
Multidimensional Arrays
Multidimensional arrays are arrays of arrays. These, as the name suggests, are arrays that contain other arrays, which allows us to create complex data structures. The size of the array is not always known or fixed, and can be dynamically allocated.
Dynamic Arrays
Dynamic arrays are arrays that can be resized during runtime. This is a very powerful feature, as it allows programmers to adapt to the data they are processing. Languages like Python and JavaScript support dynamic arrays out of the box, while in languages like C and Java, dynamic arrays must be manually implemented.
Array Operations
There are several operations that can be performed on arrays, such as:
- Traversing: print all the array elements one by one. - Insertion: Adds an element at the given index. - Deletion: Deletes an element at the given index. - Search: Searches an element using the given index or by the value. - Update: Updates an element at the given index.
Applications of Arrays
Arrays are used in various ways in programming. Here are a few examples:
- To implement mathematical vectors and matrices. - To implement other data structures like stacks, queues, heaps, hash tables, and trees. - To implement dynamic programming solutions. - In databases, to store multiple rows with the same structure.
See Also
- Data Structure - Dynamic Array - Multidimensional Array - Matrix (Mathematics) - List (Abstract Data Type)