Dynamic Array
Introduction
A dynamic array is a data structure in computer science that allows for random access and variable size. Unlike a static array, which has a fixed size, a dynamic array can grow or shrink as needed to accommodate the addition or removal of elements. This flexibility is achieved through dynamic memory allocation, a process that assigns memory during the execution of a program.
Structure and Operation
A dynamic array is typically implemented using a static array, which serves as a buffer. The buffer has an initial capacity, and when this capacity is exceeded, the array is resized. This is done by creating a new, larger array and copying the elements from the old array to the new one. This process is known as reallocation.
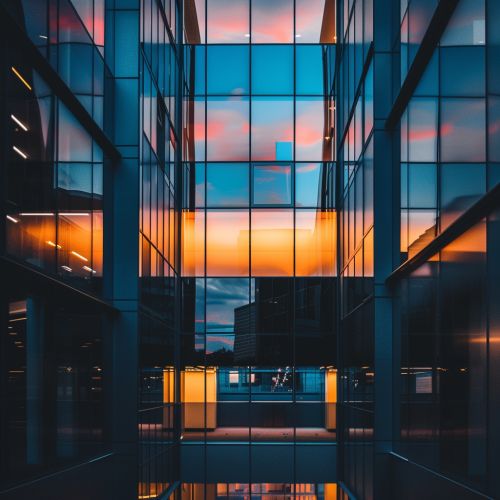
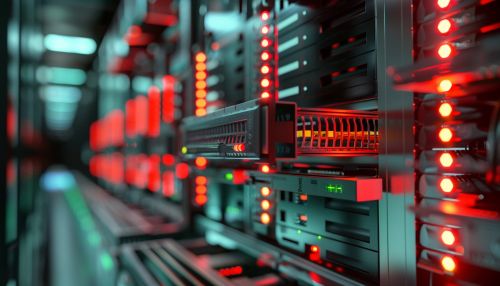
The size of a dynamic array refers to the number of elements it currently holds, while its capacity refers to the maximum number of elements it can hold without resizing. The capacity is always greater than or equal to the size. When elements are added to a dynamic array, its size increases. If the size exceeds the capacity, the array is resized. Conversely, when elements are removed, the size decreases. If the size falls significantly below the capacity, the array may be resized to a smaller capacity to conserve memory.
Dynamic Array Operations
Dynamic arrays support several fundamental operations, including:
- Access: The elements of a dynamic array can be accessed directly using an index. This operation is constant time, denoted as O(1), because it takes the same amount of time regardless of the size of the array.
- Insertion: Adding an element to a dynamic array can be done at the end in constant time, as long as the array's capacity is not exceeded. If the capacity is exceeded, the array must be resized, which takes linear time, denoted as O(n), where n is the number of elements in the array.
- Deletion: Removing an element from the end of a dynamic array takes constant time. Removing an element from elsewhere in the array requires shifting the subsequent elements to fill the gap, which takes linear time.
- Search: Finding an element in a dynamic array requires checking each element in turn, which takes linear time.
Implementation Details
Dynamic arrays are a fundamental component of many programming languages. For example, in C++, the standard library provides a dynamic array implementation known as 'vector'. In Java, dynamic arrays are implemented as 'ArrayList', and in Python, they are implemented as 'list'.
When implementing a dynamic array, it is important to consider the growth factor, which determines how much the capacity increases during a resize operation. A common strategy is to double the capacity each time. This approach ensures that the average time for insertion at the end is constant, a property known as amortized constant time.
Advantages and Disadvantages
Dynamic arrays offer several advantages over static arrays. They can adjust their size to match the number of elements, which can conserve memory. They also provide efficient access to elements and can be resized as needed.
However, dynamic arrays also have some disadvantages. The need to occasionally resize the array can lead to performance issues, particularly for large arrays. Additionally, because elements are stored in contiguous memory, insertion and deletion operations can be costly, as they may require shifting many elements.
Applications
Dynamic arrays are used in a wide range of applications. They are often used to implement other data structures, such as stacks, queues, and hash tables. They are also used in many algorithms that require a variable-size list of elements.