Data type
Introduction
A data type is a classification of data which tells the compiler or interpreter how the programmer intends to use the data. Most programming languages support basic data types of integer numbers (of varying sizes), floating-point numbers (which approximate real numbers), characters and Booleans. A data type provides a set of values from which an expression (i.e. variable, function, etc.) may take its values.
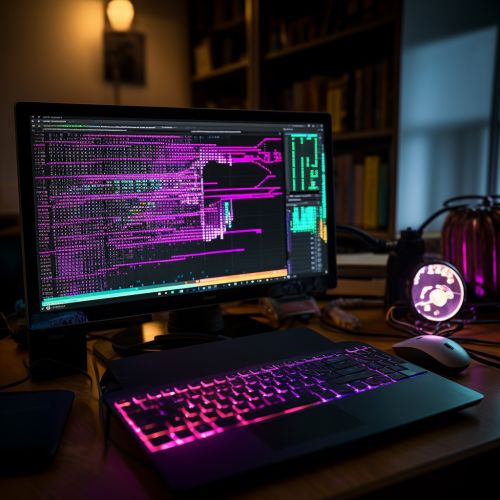
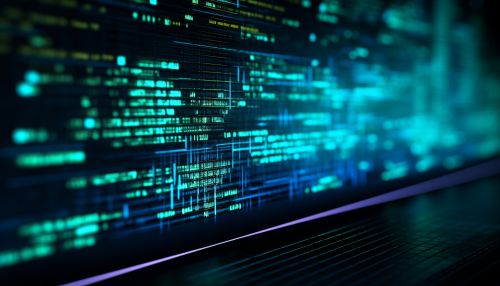
Primitive Data Types
Primitive data types are the most basic data types available within a programming language. These types serve as the building blocks for data manipulation in any language. Examples of primitive data types include integers, floating point numbers, characters, and Booleans.
Integer
An integer in computer science is a datum of integral data type, a data type that represents some range of mathematical integers. Integral data types may be of different sizes and may or may not be allowed to contain negative values.
Floating Point
A floating point type variable is a variable that can hold a real number, such as 4320.0, -3.33, or 0.01226. Floating-point numbers are often used to approximate analog and continuous values because they have greater resolution than integer types.
Character
A character data type is a primitive type that includes any alphanumeric character that can be used in text. This includes all the uppercase and lowercase alphabets, digits, punctuation marks, etc.
Boolean
A Boolean data type has one of two possible values (usually denoted true and false), intended to represent the two truth values of logic and Boolean algebra.
Non-Primitive Data Types
Non-primitive data types, also known as reference or object data types, are more complex types of data. They don't just store a value, but rather a collection of values in various formats.
Array
An array is a data structure consisting of a collection of elements (values or variables), each identified by at least one array index or key. An array is stored such that the position of each element can be computed from its index tuple by a mathematical formula.
String
A string is a data type used in programming, such as an integer and floating point unit, but is used to represent text rather than numbers. It is comprised of a set of characters that can also contain spaces and numbers.
List
A list or sequence is an abstract data type that represents a countable number of ordered values, where the same value may occur more than once.
Dictionary
A dictionary (also known as a map) is an abstract data type composed of a collection of (key, value) pairs, such that each possible key appears at most once in the collection.
Type Systems
A type system is a logical system comprising a set of rules that assigns a property called a type to the various constructs of a computer program, such as variables, expressions, functions or modules.
Static Typing
Static typing is a programming language feature in which type checking is performed during compile-time as opposed to run-time.
Dynamic Typing
Dynamic typing is a type system in which variables are not required to have their type defined prior to being assigned a value.
Conclusion
Understanding data types and how they function within a programming language is crucial for any programmer or computer scientist. They form the foundation of any data manipulation, and a deep understanding of these types can greatly improve the efficiency and effectiveness of your code.