String (computer science)
Definition
In computer science, a string is a sequence of characters. A character is a symbol that represents an alphabetic letter, numeric digit, punctuation mark, or other symbol. Strings are a fundamental concept in computer science and are used in almost all areas of programming and data manipulation.
History
The concept of strings in computer science has its roots in the early days of computers. The first computers used binary code to represent data, but this was cumbersome and difficult to work with. As computers evolved, the need for a more human-readable form of data representation became apparent, leading to the development of character sets like ASCII and EBCDIC. These character sets allowed data to be represented as strings of characters, making it easier for humans to interact with computers.
String Representation
In most programming languages, strings are represented as arrays of characters. This means that a string is essentially a list of characters, stored in a specific order. For example, the string "Hello" is represented as an array of five characters: 'H', 'e', 'l', 'l', and 'o'.
Each character in a string is associated with an index, which is a numerical value that represents the position of the character in the string. In most languages, the index of the first character is 0, the index of the second character is 1, and so on.
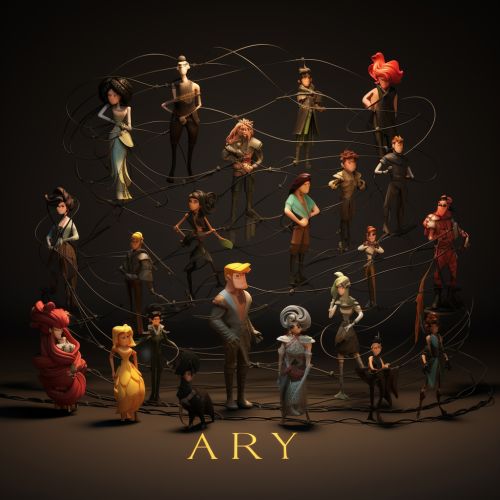
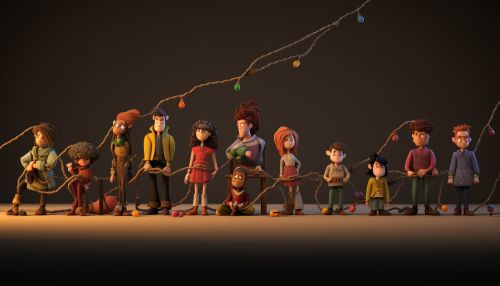
String Operations
There are several fundamental operations that can be performed on strings. These include:
- Concatenation: This is the operation of joining two strings end-to-end. For example, the concatenation of the strings "Hello" and "World" results in the string "HelloWorld".
- Substring: This operation involves extracting a portion of a string. For example, the substring of "HelloWorld" from index 0 to 4 is "Hello".
- Length: This operation returns the number of characters in a string. For example, the length of the string "HelloWorld" is 10.
- Comparison: Strings can be compared to determine if they are equal, or to determine their lexicographic order. This is often done using ASCII values of the characters.
- Search: This operation involves finding the position of a substring within a string. For example, in the string "HelloWorld", the substring "World" starts at index 5.
String Data Types
In many programming languages, strings are a distinct data type. This means that they have special properties and operations that are not available to other types of data. For example, in the Java programming language, strings are an immutable data type, which means that once a string is created, it cannot be changed. Instead, any operation that appears to modify a string actually creates a new string.
Some languages, like C, do not have a built-in string data type. Instead, strings are represented as arrays of characters, and string operations are performed using functions from the standard library.
String Encoding
Strings are typically encoded using a character encoding scheme, which maps each character in the string to a specific binary representation. The most common encoding scheme is ASCII, which represents each character as a 7-bit binary number. However, ASCII only supports 128 different characters, which is not enough to represent all the characters used in various languages around the world.
To support a wider range of characters, other encoding schemes like Unicode are used. Unicode can represent over a million different characters, making it suitable for representing text in virtually any language.
String Interpolation
String interpolation is a process in programming where variables are inserted into a string. This is useful for creating dynamic strings where some parts of the string are determined at runtime. The syntax for string interpolation varies between programming languages, but typically involves enclosing the variable in some form of delimiter within the string.
String in Different Programming Languages
Different programming languages handle strings in different ways. For example, in Python, strings are a built-in data type and have a variety of built-in methods for manipulation. In contrast, in languages like C, strings are simply arrays of characters and manipulation requires explicit use of functions from the standard library.