Space Complexity
Introduction
Space complexity is a concept in computer science that deals with the amount of memory used by an algorithm (including the input values to the algorithm) to execute and produce the result. It is often expressed using Big O notation, which describes the upper bound of the space complexity in the worst-case scenario.
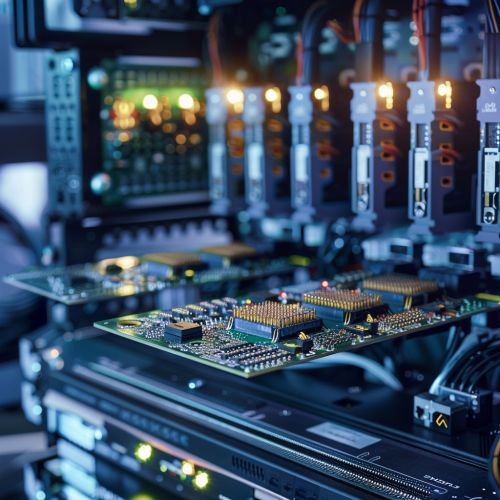
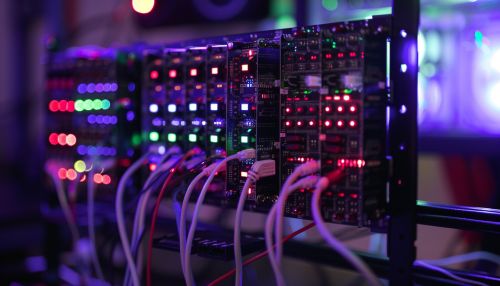
Understanding Space Complexity
In computer science, when we analyze an algorithm, we not only look at the time complexity, i.e., the amount of time an algorithm takes to run, but also at the space complexity. The space complexity of an algorithm or a computer program is the amount of memory space required to solve an instance of the computational problem as a function of characteristics of the input. It is equivalent to the maximum over inputs of the size of the computation history, where the size is measured in the number of memory cells.
Space complexity includes both the auxiliary space and the space used by the input. The auxiliary space is the extra space or the temporary space used by an algorithm. The space used by the input is the space needed to store the input itself.
Factors Affecting Space Complexity
There are several factors that affect the space complexity of an algorithm. These include:
- Input Size: The size of the input is directly proportional to the space complexity. Larger inputs require more space to store them, hence increasing the space complexity.
- Recursive Function Calls: Recursive function calls require additional space for each recursive call made. This additional space is used to save the state of the current function call so that execution can resume correctly after the recursive call is finished.
- Data Structures: The use of data structures in an algorithm also affects the space complexity. Different data structures have different memory requirements, and the choice of data structure can significantly impact the space complexity.
- Variables: The number and size of variables used in an algorithm also affect the space complexity. More variables or larger variables (such as arrays or objects) require more memory space.
Calculating Space Complexity
Space complexity is calculated by counting the maximum space needed by the algorithm. The space complexity S(P) of any computational process P is defined as the maximum auxiliary space used by the process throughout its execution.
To calculate the space complexity, we need to know the total space required by the algorithm. This includes the space required for variables, program instructions, etc.
The formula for calculating space complexity is:
S(P) = C + SP(I)
where:
- S(P) is the space complexity
- C is the fixed part (space required for the variables and simple statements, independent of the size of the problem)
- SP(I) is the variable part (space required by the variables that have a size dependent on the size of the problem)
Examples of Space Complexity
Here are some examples of space complexity for common algorithms:
- Linear Search: The space complexity of a linear search algorithm is O(1), which means it requires a constant amount of space regardless of the input size. This is because the linear search algorithm only requires a single variable to store the current item, regardless of the size of the input array.
- Recursive Fibonacci: The space complexity of a recursive Fibonacci algorithm is O(n), where n is the input number. This is because each recursive call to the function requires additional space on the stack, and there are n recursive calls in total.
- Merge Sort: The space complexity of a merge sort algorithm is O(n), where n is the size of the input array. This is because the merge sort algorithm requires an auxiliary array of the same size as the input array.
Reducing Space Complexity
Reducing space complexity can be as crucial as reducing time complexity, especially in systems with limited memory. Here are some strategies to reduce space complexity:
- Eliminate Recursion: Recursion can lead to high space complexity due to the stack space required for each recursive call. By eliminating recursion and using iteration instead, we can often reduce space complexity.
- Use Space-Efficient Data Structures: The choice of data structure can significantly impact space complexity. By choosing more space-efficient data structures, we can reduce space complexity. For example, using a bit vector instead of an array or list can save space.
- In-Place Algorithms: In-place algorithms are algorithms that require a constant amount of space to execute, regardless of the input size. By designing in-place algorithms, we can keep space complexity to a minimum.
- Space-Time Tradeoff: Sometimes, we can reduce space complexity by increasing time complexity, and vice versa. This is known as the space-time tradeoff. For example, using a lookup table can reduce time complexity but increase space complexity.
Conclusion
Space complexity is a critical aspect of algorithm analysis, especially in systems with limited memory. Understanding and optimizing space complexity can lead to more efficient algorithms and better system performance.