Out Function
Introduction
The "out" function is a term used in various programming languages, including C++, C#, and Java, to denote a method that outputs data. It is a fundamental concept in computer programming, allowing programs to interact with users or other systems by producing output.

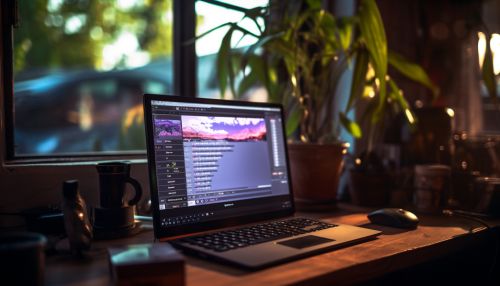
Usage in Different Programming Languages
C++
In C++, the "out" function is typically associated with output streams, particularly the cout object of the iostream library. The cout object, coupled with the insertion operator (<<), is used to output data to the standard output, which is usually the screen.
C#
In C#, the "out" keyword is used in a different context. It is used as a parameter modifier, indicating that an argument is passed by reference and is intended to be modified by the called method. This allows for multiple return values in a method, enhancing the flexibility of the language.
Java
In Java, the "out" function is part of the System class and is used for printing output to the console. The most common use is System.out.println(), which prints a line of text to the console.
In-Depth Explanation
C++
In C++, the cout object is part of the standard library and is an instance of the ostream class. It is used in conjunction with the insertion operator (<<) to output data. For example, to print the string "Hello, World!" to the console, one would use the following code:
```cpp
- include <iostream>
int main() {
std::cout << "Hello, World!"; return 0;
} ```
In this example, "Hello, World!" is the operand of the << operator, and cout is the ostream object to which the string is sent.
C#
In C#, the "out" keyword is used to signify that a method should modify its argument. This is different from the "ref" keyword, which also passes an argument by reference, but requires that the argument be initialized before it is passed. The "out" keyword does not have this requirement. Here is an example of a method that uses an out parameter:
```csharp public void GetValues(out int x, out int y) {
x = 5; y = 42;
} ```
In this example, the values of x and y are set within the GetValues method.
Java
In Java, System.out is a static member field of the System class and is an instance of the PrintStream class. It has several methods for printing to the console, but the most commonly used one is println(). Here is an example:
```java public class Main {
public static void main(String[] args) { System.out.println("Hello, World!"); }
} ```
In this example, "Hello, World!" is printed to the console.