Floyd–Warshall Algorithm
Introduction
The Floyd–Warshall algorithm is a graph analysis algorithm for finding shortest paths in a weighted, directed graph. It is a dynamic programming algorithm that operates on all pairs of vertices simultaneously, providing a comprehensive solution for the shortest path problem.
Algorithm Description
The Floyd–Warshall algorithm operates by incrementally improving an estimate on the shortest path between two vertices, until the estimate is optimal. It does this by repeatedly examining all possible paths between each pair of vertices and updates the shortest distance found so far. The algorithm uses a matrix to store the distances between each pair of vertices, which is updated iteratively.
The algorithm begins by initializing the distance from each vertex to itself as 0 and the distance from each vertex to all other vertices as the weight of the edge connecting them, or infinity if there is no such edge. Then, for each vertex, it considers each possible pair of vertices and checks if the current path between them can be improved by going through the vertex under consideration. If so, it updates the distance between the pair of vertices in the matrix.
Algorithm Pseudocode
The pseudocode for the Floyd–Warshall algorithm is as follows:
``` function FloydWarshall(G):
let dist be a |V| × |V| array of minimum distances initialized to ∞ (infinity) for each vertex v in G: dist[v][v] ← 0 for each edge (u,v) in G: dist[u][v] ← w(u,v) // The weight of the edge (u,v) for each vertex k in G: for each vertex i in G: for each vertex j in G: if dist[i][j] > dist[i][k] + dist[k][j] dist[i][j] ← dist[i][k] + dist[k][j] return dist
```
Time Complexity
The time complexity of the Floyd–Warshall algorithm is O(n^3), where n is the number of vertices in the graph. This is because the algorithm performs a constant amount of work for each pair of vertices for each vertex in the graph, resulting in a cubic running time. Despite this, the algorithm is efficient for dense graphs, where the number of edges is close to the maximum possible number of edges.
Space Complexity
The space complexity of the Floyd–Warshall algorithm is O(n^2), where n is the number of vertices in the graph. This is because the algorithm requires a two-dimensional matrix of size n × n to store the distances between each pair of vertices.
Applications
The Floyd–Warshall algorithm is used in a variety of applications, including:
- In computer networking, to find the shortest path through a network. - In operations research, to solve the all-pairs shortest path problem in transportation and logistics. - In game theory, to calculate the length of a game. - In robotics, for path planning.
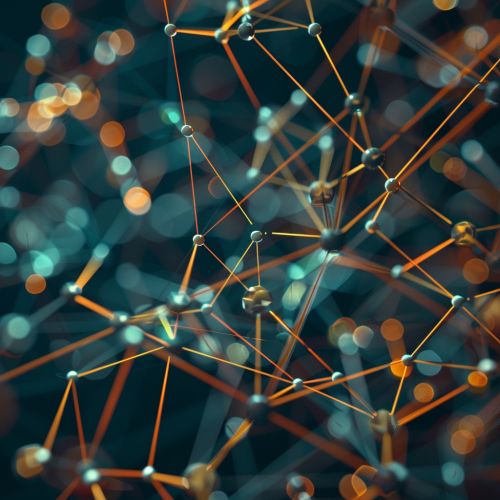
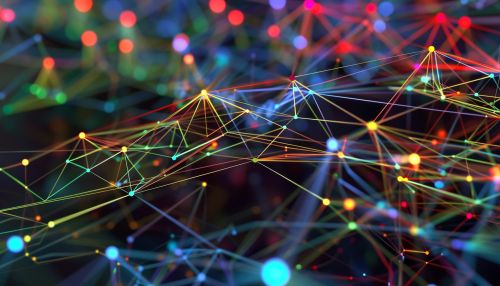
See Also
- Dijkstra's algorithm - Bellman-Ford algorithm - Johnson's algorithm - A* search algorithm