Expression Templates
Introduction
Expression templates are a sophisticated programming technique used in C++ to optimize the performance of mathematical operations on complex data structures. They leverage the power of template metaprogramming to transform expressions into efficient, inline code, reducing the overhead typically associated with operator overloading. This technique is particularly beneficial in scientific computing, where operations on large matrices or vectors are frequent and performance is critical.
Background and Motivation
The concept of expression templates arose from the need to address the inefficiencies in operator overloading. In traditional operator overloading, each operation on objects like matrices or vectors creates temporary objects, leading to unnecessary memory allocations and deallocations. This can significantly degrade performance, especially in computationally intensive applications. Expression templates solve this problem by eliminating temporary objects and enabling the compiler to generate optimized code that performs the operations directly.
How Expression Templates Work
Expression templates work by representing expressions as a tree of objects, where each node corresponds to an operation. These objects are lightweight and do not perform any computations themselves. Instead, they store references to their operands and the operation to be performed. When the final result of the expression is needed, the entire expression tree is evaluated in a single pass, directly computing the result without creating intermediate temporaries.
Template Metaprogramming
Template metaprogramming is the foundation of expression templates. It allows the creation of templates that can manipulate types and values at compile time. This capability is exploited to build expression trees that represent complex mathematical expressions. The use of templates ensures that the expression is fully resolved at compile time, allowing the compiler to generate highly optimized code.
Operator Overloading
In the context of expression templates, operator overloading is used to construct the expression tree. Each overloaded operator returns an object representing the operation, rather than performing the operation immediately. This deferred execution model is key to the efficiency of expression templates, as it postpones computation until the entire expression is known.

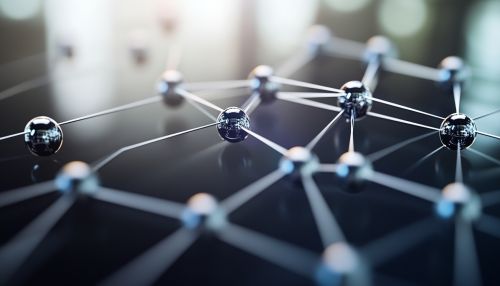
Implementation Details
The implementation of expression templates involves several key components:
Expression Classes
Expression classes are the building blocks of expression templates. Each class represents a specific operation, such as addition or multiplication, and stores references to its operands. These classes are typically lightweight and designed to be combined in a hierarchical manner to form complex expressions.
Template Specialization
Template specialization is used to handle different types of operations and operands. By specializing templates for specific operations, it is possible to optimize the evaluation of expressions for particular data types or structures. This allows for fine-tuned performance improvements in specific scenarios.
Lazy Evaluation
Lazy evaluation is a crucial aspect of expression templates. It ensures that computations are only performed when the final result is required. This approach minimizes unnecessary computations and memory usage, contributing to the overall efficiency of the technique.
Advantages of Expression Templates
Expression templates offer several advantages over traditional operator overloading:
- **Performance**: By eliminating temporary objects and enabling inline computation, expression templates significantly improve performance, particularly in applications involving large data sets.
- **Flexibility**: The use of templates allows for flexible and reusable code, which can be adapted to different types of operations and data structures.
- **Compile-time Optimization**: Expression templates leverage the compiler's optimization capabilities, resulting in highly efficient code.
Challenges and Limitations
Despite their advantages, expression templates also present certain challenges:
- **Complexity**: The implementation of expression templates can be complex, requiring a deep understanding of template metaprogramming and operator overloading.
- **Debugging Difficulty**: The use of templates can obscure the code, making debugging more challenging. Error messages can be cryptic and difficult to interpret.
- **Compiler Support**: Not all compilers fully support the advanced features required for expression templates, which can limit their portability.
Applications
Expression templates are widely used in scientific computing and numerical libraries, where performance is critical. They are particularly beneficial in applications involving:
- **Matrix and Vector Operations**: Expression templates optimize operations on large matrices and vectors, reducing computational overhead.
- **Finite Element Analysis**: In finite element analysis, expression templates can improve the efficiency of complex mathematical computations.
- **Signal Processing**: Expression templates are used in signal processing applications to optimize the performance of mathematical transformations.
Conclusion
Expression templates represent a powerful technique for optimizing mathematical computations in C++. By leveraging template metaprogramming and operator overloading, they eliminate the inefficiencies associated with temporary objects and enable the generation of highly optimized code. While they present certain challenges, their benefits in terms of performance and flexibility make them an invaluable tool in the arsenal of modern C++ programming.