Exception handling
Overview
Exception handling is a critical aspect of software development that deals with the occurrence of exceptions - anomalous or exceptional conditions requiring special processing - during the execution of a program. In computer programming, an exception is an event that occurs during the execution of a program that disrupts the normal flow of the program's instructions. Exception handling is a construct in many programming languages to handle or deal with errors automatically. Many programming languages like Java, C#, and Python have built-in support for exception handling.
Rationale
Exception handling is used to change the normal flow of the code execution if a specified error occurs. Exception handling in software involves the process of responding to the occurrence, during computation, of exceptions – anomalous or exceptional conditions requiring special processing – often changing the normal flow of program execution. This kind of processing (or, more generally, computation) can be classified into two types: hardware and software exception handling.
Hardware Exception Handling
Hardware exception handling/traps: Hardware exceptions are processed by the CPU. It is intended to support error detection and redirects the program flow to error handling service routines. The CPU's exception handling mechanism works in tandem with the operating system's exception handling module.
Software Exception Handling
Software exception handling and the support provided by software tools differs somewhat from what is understood by exception in hardware, but similar concepts are involved. In software, an exception is a construct to handle program anomalies. Software exception handling includes support for exception raising (also called exception throwing), an action performed by a program or the runtime system, which initiates the exception handling process.
Exception Handling Models
There are several models of exception handling that programming languages use. These include termination models, resumption models, and hybrid models.
Termination Model
In the termination model of exception handling, the program's control flow is transferred from the point where the exception was raised to an exception handler, and the computation is terminated. Languages that support this model include Ada, Java, Modula-3, ML, OCaml, Python, and Ruby.
Resumption Model
In the resumption model of exception handling, the exception handler is responsible for correcting the problem that caused the exception and then control is transferred back to the point where the exception was raised. Languages that support this model include Common Lisp, PL/I, CLU, and Smalltalk.
Hybrid Model
The hybrid model of exception handling is a combination of the termination and resumption models. In this model, after the exception is raised, control is transferred to the exception handler. However, the handler may decide whether to terminate the computation or to resume it from the point where the exception was raised. Languages that support this model include C++ and C#.
Exception Handling Mechanisms
Different programming languages have different methods and features for handling exceptions. For example, in some languages, like Java and C#, exceptions are handled using a try-catch-finally construct. Other languages, like Python, use a try-except-finally construct.
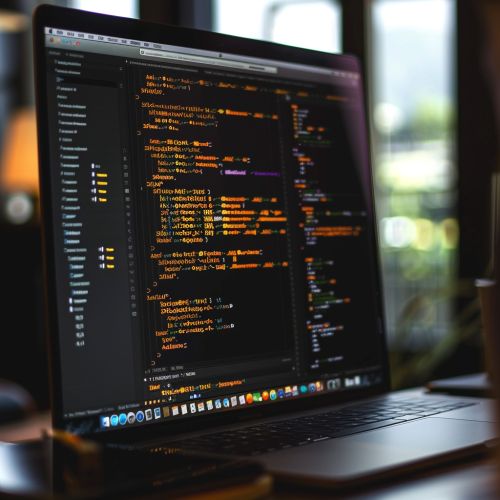
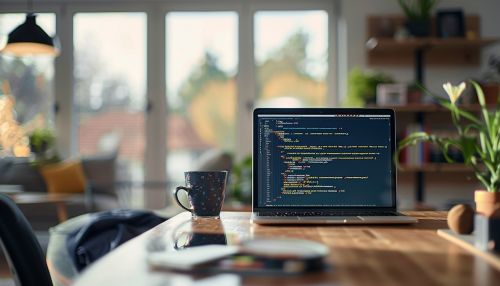
Try-Catch-Finally
In this mechanism, the code that might throw an exception is placed inside the 'try' block. If an exception occurs, the flow of control is transferred to a 'catch' block that handles the exception. The 'finally' block consists of code that is always executed after the 'try' and 'catch' blocks, regardless of whether an exception was thrown or caught.
Try-Except-Finally
This mechanism is similar to the try-catch-finally mechanism, but it is used in languages like Python that use the keywords 'try', 'except', and 'finally'. The 'try' block contains the code that might throw an exception, the 'except' block contains the code to handle the exception, and the 'finally' block contains code that is always executed, regardless of whether an exception was thrown or caught.
Best Practices in Exception Handling
Exception handling is a crucial part of software development. Here are some best practices that developers should follow:
- Use exceptions for exceptional conditions: Exceptions should be used to signal exceptional conditions that the code cannot handle, not to control regular program flow.
- Catch specific exceptions: Catching all exceptions can make it difficult to debug the program. Instead, catch specific exceptions that you expect might occur.
- Don't swallow exceptions: If you catch an exception, handle it. Don't just catch it and do nothing.
- Use finally blocks: Finally blocks should be used to clean up resources, like closing a file or a database connection, regardless of whether an exception was thrown or not.
- Document exceptions: If a method can throw an exception, document it using comments or, in languages that support it, the 'throws' keyword.
See Also
- Software Development - Programming Languages - Java - Python - C#