Do while loop
Introduction
A do while loop is a control flow statement in programming that executes a block of code at least once, and then repeatedly executes the block, or not, depending on a given boolean condition at the end of the block. The do while loop is a variant of the while loop. This type of loop is known as a post-test loop because its condition is checked after the loop's body is executed.
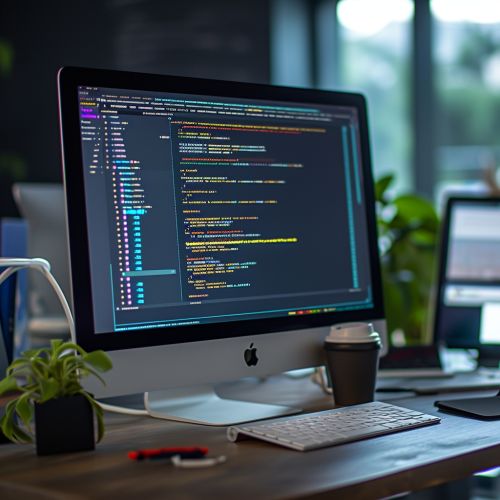
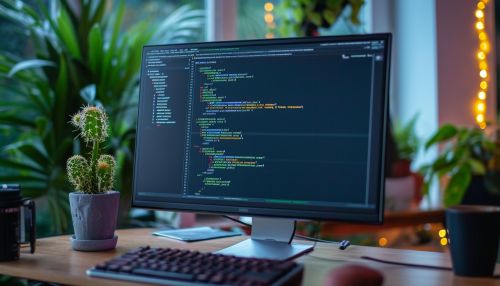
Syntax
The basic syntax of a do while loop in most programming languages is as follows:
``` do {
// Statements
} while(condition); ```
In this structure, the condition is evaluated after the execution of the statements within the loop. Therefore, the statements within the loop are executed at least once, even if the condition is false.
Working of Do While Loop
The do while loop works in the following manner:
1. The statements inside the loop are executed. 2. The condition is evaluated. If the condition is true, the flow of control jumps back up to do, and the statements in the loop execute again. This process continues until the given condition returns false. 3. When the condition becomes false, the control comes out of the loop and jumps to the next statement in the program after the do while loop.
Comparison with While Loop
A do while loop is similar to a while loop, but with a critical difference. In a while loop, the condition to be evaluated is checked at the beginning of the loop. If the condition is false at the start, the loop will never be executed. On the other hand, in a do while loop, the condition is checked at the end, which ensures that the loop is executed at least once, regardless of the condition.
Use Cases
Do while loops are used when the statements within the loop need to be executed at least once before checking the condition. They are commonly used when the exact number of iterations is not known in advance. Here are some use cases:
- Reading user input: A do while loop can be used to read the user input and then check whether it meets certain conditions. - Menu driven programs: In these programs, a list of options is displayed to the user, and the user is allowed to select an option. The program will continue to display these options until the user selects the option to quit.
Advantages and Disadvantages
Advantages of do while loop:
- It executes the loop body at least once, which is useful in scenarios where the statements need to be executed before the condition is tested. - It simplifies the program structure and makes it easier to read and understand.
Disadvantages of do while loop:
- It can lead to an infinite loop if the condition never becomes false. - It is not suitable for situations where the condition needs to be checked before the loop body is executed.
Examples
Here are some examples of do while loops in various programming languages:
C++
```cpp
- include<iostream>
using namespace std; int main() {
int i = 1; do { cout<<i<<endl; i++; } while(i <= 10); return 0;
} ```
Java
```java public class DoWhileExample {
public static void main(String args[]){ int i=1; do{ System.out.println(i); i++; } while(i<=10); }
} ```
Python
In Python, there is no do while loop. However, we can create a similar loop using the while loop like this:
```python i = 1 while True:
print(i) i += 1 if(i > 10): break
```
See Also
- For loop - While loop - Control flow - Programming language