Debugging
Introduction
Debugging is the process of identifying and resolving problems or defects within a computer program. It involves a systematic process of finding and reducing the number of bugs, or defects, in a computer program, making it behave as expected. Debugging tends to be harder when various subsystems are tightly coupled, as changes in one may cause bugs to emerge in another.
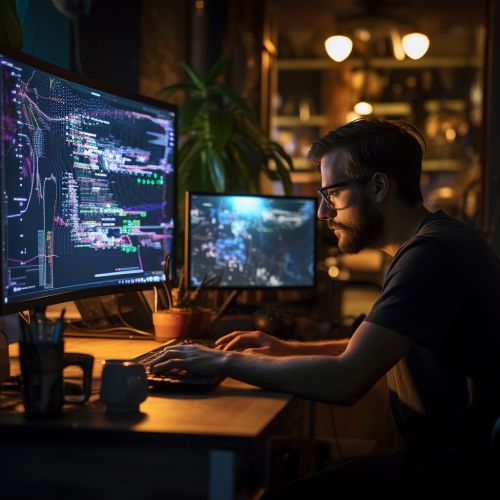
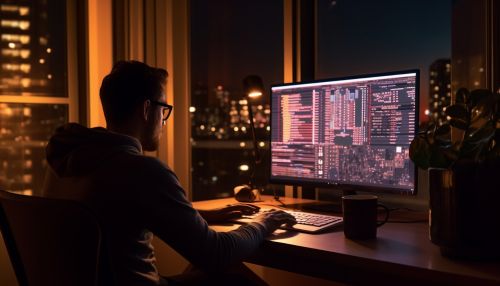
History of Debugging
The concept of debugging originated in the early days of computers. The term "bug" was used in an account by computer pioneer Grace Murray Hopper, who publicized the cause of a malfunction in an early electromechanical computer. A typical version of the story is given by this quote regarding an incident in 1947. The operators traced an error in the Mark II to a moth trapped in a relay, coining the term bug. This bug was carefully removed and taped to the log book. Since then, the term "debugging" has been used to refer to the process of removing errors in computer code.
Debugging Techniques
There are several techniques for debugging an application or system. These include, but are not limited to:
Interactive Debugging
Interactive debugging is the most common debugging technique. This involves the use of debugging tools or debuggers such as GNU Debugger (GDB) or Visual Studio Debugger. These tools allow the programmer to monitor the execution of a program, stop it, restart it, set breakpoints, and change values in memory. The debugger can also be used to examine the stack, a special area of computer memory where information is stored about the active subroutines of the computer program.
Print Debugging
Print debugging (or tracing) is the act of watching (live or recorded) trace statements, or print statements, that indicate the flow of execution of a process. This is sometimes called "printf debugging," due to the use of the printf function in C. This kind of debugging was turned on by the command TRON in the original versions of the novice-oriented BASIC programming language. TRON stood for, "Trace On." TRON caused the line numbers of each BASIC command line to print as the program ran.
Remote Debugging
Remote debugging is the process of debugging a program running on a system different from the debugger. To start remote debugging, a debugger connects to a remote system over a network. The debugger can then control the execution of the program on the remote system and retrieve information about its state.
Post-mortem Debugging
Post-mortem debugging is debugging of the program after it has already crashed. Tools used for post-mortem debugging include core dumps and crash dumps. These files can be analyzed to determine what program state led to the crash.
"Wolf Fence" Algorithm
The "Wolf Fence" Algorithm is a debugging algorithm used for finding the section of code where the error is. In this method, one first isolates the section of code where the bug likely resides (the "wolf"), then inserts a check (the "fence") that verifies that the wolf is indeed within the fence. This is repeated, with smaller and smaller sections of code, until the specific line containing the bug is identified.
Debugging Process
The debugging process starts with an attempt to reproduce the reported problem. Once the problem has been reproduced, the input of the program may need to be simplified to make it easier to debug. For example, a bug in a compiler can make it crash when parsing some large source file. However, after simplification of the test case, only few lines from the original source file can be sufficient to reproduce the same crash. Such simplification can be made manually, using a divide-and-conquer approach.
After the test case is sufficiently simplified, a programmer can use a debugger tool to examine program states (values of variables, plus the call stack) and track down the origin of the problem(s). Alternatively, tracing can be used. In simple cases, tracing is just a few print statements, which output the values of variables at certain points of program execution.
Debugging Tools
Debugging tools (often called debuggers) are used to identify coding errors at various development stages. Debuggers offer features such as running a program step by step (single-stepping or program animation), stopping (breaking) (pausing the program to examine the current state) at some event or specified instruction by means of a breakpoint, and tracking the values of some variables. Some debuggers offer two modes of operation – full or partial simulation – to limit this impact.
Debugging Strategies
Debugging is a methodical process of finding and reducing the number of bugs, or defects, in a computer program or a piece of electronic hardware, thus making it behave as expected. Debugging tends to be harder when various subsystems are tightly coupled, as changes in one may cause bugs to emerge in another.
There are many debugging strategies:
Brute Force
This is the most basic debugging strategy and involves looking at all the code until the bug is found. This is often the last resort when all other debugging techniques have failed.
Backtracking
This is a very common and simple debugging technique where the programmer works backwards from the point of failure to the last point where the program was working correctly.
Cause Elimination
This strategy involves making hypotheses about the cause of a bug and testing them. This is often done by process of elimination – eliminating potential causes of a bug until you’re left with the actual cause.
Debugging in Various Programming Languages
Debugging tactics can vary by the programming language being used and the nature of the program itself. For example, debugging a Python script may involve different approaches and tools compared to debugging a Java application. Similarly, debugging a single-threaded application in C++ differs from debugging a multithreaded application in the same language.
Conclusion
Debugging is an essential part of the software development process. Despite the complexity and the potential for frustration, debugging is a rewarding process that leads to more stable and reliable software. With a variety of techniques and tools available, programmers have a range of strategies to tackle bugs in their code.
See Also
- Software Testing - Software Development Process - Programming Paradigms