Class diagram
Overview
A class diagram is a type of static structure diagram that is used in software engineering to describe the structure of a system by showing its classes, attributes, operations, and the relationships among objects. It is a central part of the Unified Modeling Language (UML) and is widely used in object-oriented programming. Class diagrams are essential tools for both the design and documentation of software systems.
Components of a Class Diagram
Class diagrams consist of several key components:
Classes
Classes are the fundamental building blocks of a class diagram. Each class is represented by a rectangle divided into three sections: the top section contains the class name, the middle section contains the attributes, and the bottom section contains the methods or operations.
Attributes
Attributes are the properties or characteristics of a class. They are usually listed in the middle section of the class rectangle. Each attribute has a name and a type, and can also have visibility indicators such as public (+), private (-), or protected (#).
Operations
Operations, also known as methods, are the functions or behaviors that a class can perform. They are listed in the bottom section of the class rectangle. Each operation has a name, a return type, and may have parameters.
Relationships
Relationships between classes are depicted using different types of lines and arrows:
- **Association**: A solid line connecting two classes, indicating a relationship between them.
- **Aggregation**: A hollow diamond at the end of a line, indicating a whole-part relationship.
- **Composition**: A filled diamond at the end of a line, indicating a stronger whole-part relationship where the part cannot exist independently of the whole.
- **Inheritance**: A solid line with a closed, unfilled arrowhead, indicating that one class is a subclass of another.
- **Dependency**: A dashed line with an open arrowhead, indicating that one class depends on another.
Detailed Analysis of Class Diagram Elements
Class Names
Class names are typically written in CamelCase and should be descriptive of the entity they represent. For example, a class representing a customer in a retail system might be named "Customer".
Attributes in Depth
Attributes can have various properties, including:
- **Visibility**: Determines who can access the attribute. Common visibility indicators are public (+), private (-), and protected (#).
- **Type**: Specifies the data type of the attribute, such as integer, string, or boolean.
- **Multiplicity**: Indicates how many instances of the attribute can exist. For example, a class might have a list of phone numbers.
Operations in Depth
Operations can also have various properties:
- **Visibility**: Similar to attributes, operations can be public, private, or protected.
- **Return Type**: Specifies the type of value the operation returns.
- **Parameters**: Operations can take one or more parameters, each with a specified type.
Associations
Associations can be further detailed with:
- **Multiplicity**: Indicates the number of instances of one class that can be associated with an instance of another class. Common multiplicities are one-to-one, one-to-many, and many-to-many.
- **Role Names**: Descriptive names given to the ends of an association to clarify the role of each class in the relationship.
Aggregation and Composition
Aggregation and composition are special types of associations that represent whole-part relationships. In aggregation, the part can exist independently of the whole, while in composition, the part cannot exist without the whole.
Inheritance
Inheritance allows one class to inherit the attributes and operations of another class. This promotes code reuse and establishes a hierarchical relationship between classes.
Dependency
Dependencies indicate that one class relies on another class to function. This is often used to show that a class uses another class as a parameter in one of its operations.
Advanced Concepts in Class Diagrams
Abstract Classes
An abstract class cannot be instantiated and is often used as a base class for other classes. Abstract classes are typically italicized in class diagrams.
Interfaces
Interfaces define a contract that other classes can implement. They are depicted as a rectangle with the keyword «interface» above the class name.
Stereotypes
Stereotypes are used to extend the UML language and add additional semantics to class diagrams. They are enclosed in guillemets (« ») and placed above the class name.
Constraints
Constraints are conditions or restrictions expressed in natural language text or in a machine-readable language for the purpose of declaring some of the semantics of an element. They are often written in curly braces ({}) and placed near the relevant element.
Packages
Packages are used to group related classes together. They are depicted as a folder-like rectangle and can contain other packages or classes.
Practical Applications of Class Diagrams
Class diagrams are used in various stages of software development:
Requirement Analysis
During requirement analysis, class diagrams help in understanding the domain model and identifying the key entities and their relationships.
System Design
In system design, class diagrams are used to define the structure of the system, including the classes, their attributes, operations, and the relationships between them.
Implementation
During implementation, class diagrams serve as a blueprint for coding. They help developers understand the structure of the system and the interactions between different classes.
Documentation
Class diagrams are also used for documenting the system. They provide a visual representation of the system's structure, making it easier for new developers to understand the system.
Best Practices for Creating Class Diagrams
Keep It Simple
Avoid adding too many details to the class diagram. Focus on the key classes and their relationships.
Use Consistent Naming Conventions
Use consistent naming conventions for classes, attributes, and operations to make the diagram easier to understand.
Group Related Classes
Use packages to group related classes together. This makes the diagram more organized and easier to navigate.
Validate the Diagram
Regularly validate the class diagram to ensure it accurately represents the system and meets the requirements.
Example of a Class Diagram
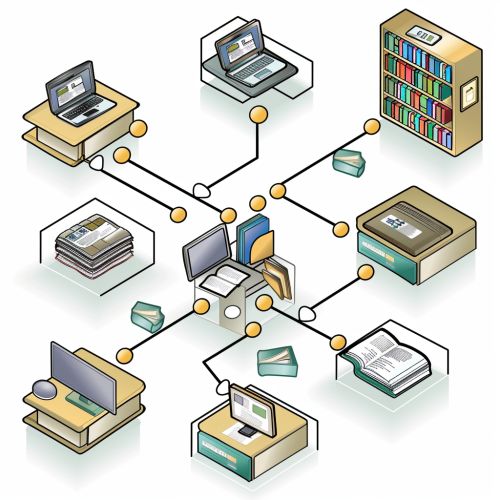
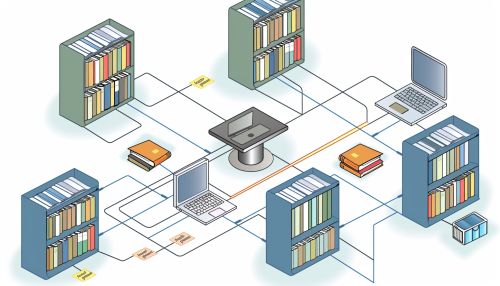