Breadth-First Search
Introduction
Breadth-first search (BFS) is a strategy for searching in a graph data structure where vertices are explored breadthwise. It is one of the simplest algorithms for searching a graph and the archetype for many important graph algorithms.
Algorithm Description
Breadth-first search starts from a root node and visits nodes in a level by level manner (i.e., visiting the ones closest to the root first). It uses a queue data structure to keep track of the nodes to be explored.
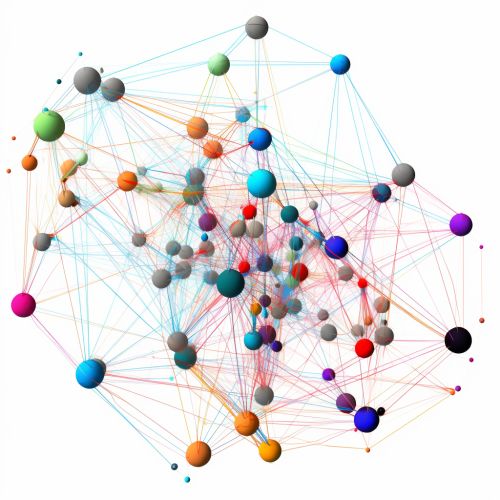
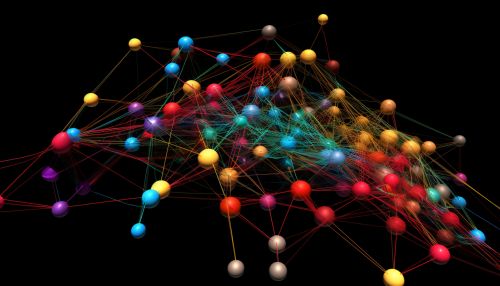
The algorithm is as follows:
1. Start by putting any one of the graph's vertices at the back of a queue. 2. Take the front item of the queue and add it to the visited list. 3. Create a list of that vertex's adjacent nodes. Add the ones which aren't in the visited list to the back of the queue. 4. Keep repeating steps 2 and 3 until the queue is empty.
Properties
Breadth-first search has several properties that distinguish it from other graph traversal algorithms:
- BFS computes the shortest path to a target node in an unweighted graph. - BFS produces a so-called 'breadth-first tree' with root s that contains all reachable vertices. - BFS can be used to determine the connected components of an unweighted graph.
Applications
Breadth-first search has a wide range of applications in computer science and related fields:
- In computer networking, BFS is used in broadcasting or multicasting where the goal is to reach all nodes. - BFS is used in garbage collection algorithms to find all objects that can be reached directly or indirectly from the root. - In Artificial Intelligence, BFS is used in uninformed search algorithms where the goal is to find a solution based on the given states and actions.
Complexity
The time complexity of BFS is O(V + E), where V is the number of vertices and E is the number of edges in the graph. This is because every vertex and every edge will be explored in the worst case. The space complexity is O(V), as in the worst case all vertices could be in the queue at the same time.
Variants
There are several variants of the breadth-first search algorithm:
- Bidirectional search: This variant of BFS starts the search from both the source and the target and stops when the two meet in the middle. - Iterative deepening depth-first search (IDDFS): This variant uses BFS to limit the search depth, then applies depth-first search within each level.
See Also
- Depth-first search - Dijkstra's algorithm - A* search algorithm