Algorithmic programming
Introduction
Algorithmic programming refers to the process of designing and implementing algorithms to solve specific computational tasks. Algorithms are step-by-step procedures for accomplishing tasks or solving problems, and they form the backbone of all computer programs. Algorithmic programming is a fundamental aspect of computer science, software engineering, and related fields.
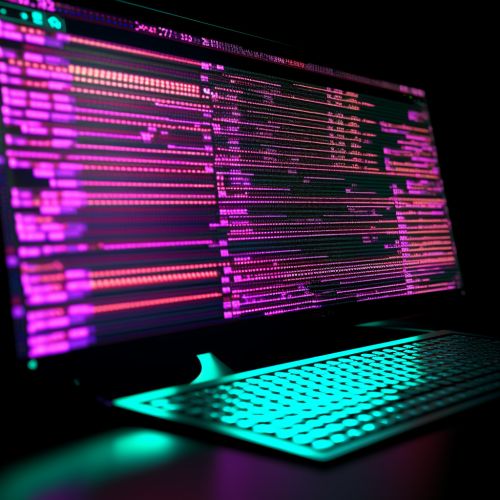
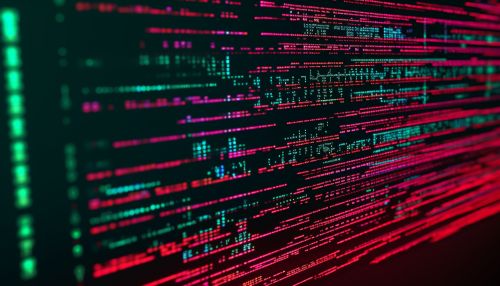
Understanding Algorithms
An algorithm is a finite sequence of well-defined, computer-implementable instructions, typically to solve a class of problems or to perform a computation. Algorithms are always unambiguous and are used as specifications for performing calculations, data processing, automated reasoning, and other tasks.
As an effective method, an algorithm can be expressed within a finite amount of space and time, and in a well-defined formal language for calculating a function. Starting from an initial state and initial input (perhaps empty), the instructions describe a computation that, when executed, proceeds through a finite number of well-defined successive states, eventually producing "output" and terminating at a final ending state. The transition from one state to the next is not necessarily deterministic; some algorithms, known as randomized algorithms, incorporate random input.
Designing Algorithms
The process of designing algorithms involves several stages. It typically begins with problem definition, where the task to be accomplished is clearly described. This is followed by the development of a model, which represents the problem in mathematical or logical terms. The algorithm is then designed using this model. The algorithm must be tested and debugged before it can be implemented in a programming language and used in a computer program.
The design of algorithms is part of the larger field of algorithmic theory. This field also includes the study of algorithm complexity and optimality. Algorithm complexity refers to the amount of resources (such as time or space) that an algorithm requires. Optimality refers to the question of whether an algorithm is the best possible solution to a problem, given the constraints.
Types of Algorithms
There are many types of algorithms, each with its own characteristics and uses. Some of the most common types include:
- Divide and Conquer Algorithms: These algorithms solve a problem by breaking it down into smaller subproblems. The solutions to the subproblems are then combined to solve the original problem.
- Greedy Algorithms: These algorithms make the locally optimal choice at each stage with the hope of finding a global optimum.
- Dynamic Programming Algorithms: These algorithms solve complex problems by breaking them down into simpler overlapping subproblems, storing the solutions to each subproblem to avoid solving the same problem multiple times.
- Backtracking Algorithms: These algorithms try out all possible solutions and discard those that do not meet the requirements.
- Brute Force Algorithms: These algorithms try all possible solutions until the correct one is found.
Implementing Algorithms in Programming Languages
Once an algorithm has been designed, it can be implemented in a programming language. This involves translating the steps of the algorithm into the syntax of the programming language. The resulting code can then be compiled and executed to perform the task specified by the algorithm.
Different programming languages offer different features and capabilities, and the choice of language can affect the implementation of an algorithm. For example, some languages offer powerful data structures that can simplify the implementation of certain algorithms. Others offer features such as recursion, which can be useful for implementing algorithms that involve repeated or nested operations.
Algorithmic Programming in Practice
In practical applications, algorithmic programming is used in a wide variety of fields. In computer science and software engineering, algorithms form the basis of all software applications, from operating systems to web browsers. In data analysis and machine learning, algorithms are used to process and analyze large datasets. In bioinformatics, algorithms are used to analyze genetic data and predict protein structures. In finance, algorithms are used to predict market trends and execute trades.