Art of Computer Programming: Difference between revisions
(Created page with "== Introduction == The art of computer programming is a comprehensive and intricate field that encompasses various techniques, methodologies, and paradigms used to write efficient and effective computer programs. This article delves into the depths of computer programming, exploring its history, fundamental concepts, advanced techniques, and the tools used by programmers. It also examines the theoretical underpinnings and practical applications of programming. == Histor...") |
No edit summary |
||
Line 29: | Line 29: | ||
Functional programming is a paradigm that treats computation as the evaluation of mathematical functions and avoids changing state and mutable data. This paradigm emphasizes the use of [[pure functions]], [[higher-order functions]], and [[recursion]]. Languages such as [[Haskell (programming language)|Haskell]] and [[Lisp (programming language)|Lisp]] are well-known for their functional programming capabilities. | Functional programming is a paradigm that treats computation as the evaluation of mathematical functions and avoids changing state and mutable data. This paradigm emphasizes the use of [[pure functions]], [[higher-order functions]], and [[recursion]]. Languages such as [[Haskell (programming language)|Haskell]] and [[Lisp (programming language)|Lisp]] are well-known for their functional programming capabilities. | ||
[[Image:Detail-93079.jpg|thumb|center|A programmer writing code on a computer screen.|class=only_on_mobile]] | |||
[[Image:Detail-93080.jpg|thumb|center|A programmer writing code on a computer screen.|class=only_on_desktop]] | |||
== Advanced Techniques == | == Advanced Techniques == |
Latest revision as of 23:14, 21 June 2024
Introduction
The art of computer programming is a comprehensive and intricate field that encompasses various techniques, methodologies, and paradigms used to write efficient and effective computer programs. This article delves into the depths of computer programming, exploring its history, fundamental concepts, advanced techniques, and the tools used by programmers. It also examines the theoretical underpinnings and practical applications of programming.
History of Computer Programming
The history of computer programming dates back to the early 19th century with the work of Ada Lovelace, who is often considered the first computer programmer. Lovelace worked on Charles Babbage's Analytical Engine, creating an algorithm for the machine to compute Bernoulli numbers. This marked the beginning of the programming era.
In the mid-20th century, the development of electronic computers led to the creation of the first high-level programming languages. Fortran, developed in the 1950s, was one of the first widely used high-level languages, designed for scientific and engineering applications. COBOL, another early language, was developed for business data processing.
The 1970s saw the emergence of C, a powerful and flexible language that became the foundation for many modern languages. The development of object-oriented programming in the 1980s, with languages like Smalltalk and C++, revolutionized software development by promoting code reuse and modularity.
Fundamental Concepts
Algorithms
An algorithm is a step-by-step procedure for solving a problem or performing a task. Algorithms are the foundation of computer programming, and their design and analysis are critical to creating efficient programs. Key concepts in algorithm design include time complexity and space complexity, which measure the efficiency of an algorithm in terms of time and memory usage, respectively.
Data Structures
Data structures are ways of organizing and storing data to enable efficient access and modification. Common data structures include arrays, linked lists, stacks, queues, trees, and hash tables. Each data structure has its own strengths and weaknesses, and the choice of data structure can significantly impact the performance of a program.
Control Structures
Control structures are constructs that dictate the flow of execution in a program. The primary control structures are sequence, selection, and iteration. Sequence refers to the execution of statements in a linear order. Selection, implemented through if-else statements and switch cases, allows for conditional execution of code. Iteration, achieved through loops such as for, while, and do-while loops, enables repeated execution of code blocks.
Programming Paradigms
Procedural Programming
Procedural programming is a paradigm based on the concept of procedure calls, where programs are composed of procedures or functions. This paradigm emphasizes a clear sequence of steps to perform tasks and is exemplified by languages like C and Pascal.
Object-Oriented Programming
Object-oriented programming (OOP) is a paradigm that organizes software design around data, or objects, rather than functions and logic. Objects are instances of classes, which encapsulate data and behavior. Key principles of OOP include encapsulation, inheritance, and polymorphism. Languages like Java and C++ are prominent examples of OOP languages.
Functional Programming
Functional programming is a paradigm that treats computation as the evaluation of mathematical functions and avoids changing state and mutable data. This paradigm emphasizes the use of pure functions, higher-order functions, and recursion. Languages such as Haskell and Lisp are well-known for their functional programming capabilities.
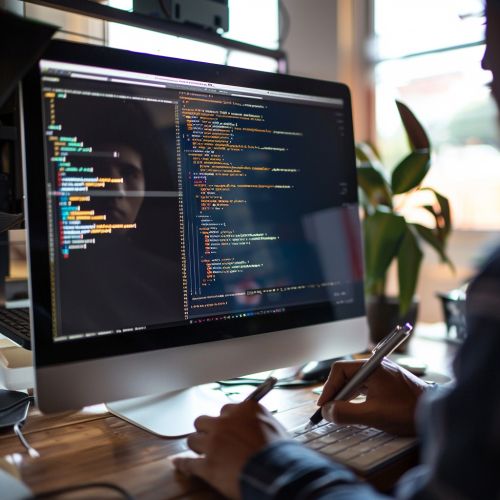
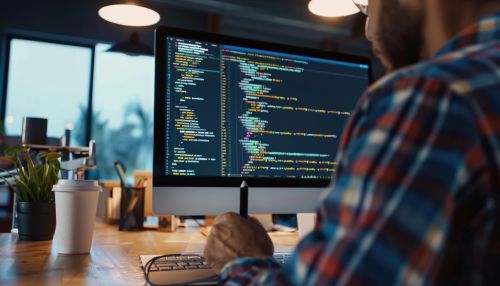
Advanced Techniques
Recursion
Recursion is a technique where a function calls itself to solve a problem. It is particularly useful for problems that can be broken down into smaller, similar subproblems. Recursion requires a base case to terminate the recursive calls and prevent infinite loops. Common applications of recursion include tree traversal and divide and conquer algorithms.
Dynamic Programming
Dynamic programming is an optimization technique used to solve complex problems by breaking them down into simpler subproblems and storing the results of these subproblems to avoid redundant computations. This technique is widely used in algorithms for shortest path, knapsack problem, and sequence alignment.
Parallel Programming
Parallel programming involves dividing a problem into smaller tasks that can be executed concurrently on multiple processors. This technique is essential for leveraging the power of modern multi-core processors and distributed computing systems. Key concepts in parallel programming include threading, synchronization, and message passing.
Tools and Environments
Integrated Development Environments (IDEs)
Integrated Development Environments (IDEs) are software applications that provide comprehensive facilities to programmers for software development. IDEs typically include a source code editor, debugger, and build automation tools. Popular IDEs include Visual Studio, Eclipse, and IntelliJ IDEA.
Version Control Systems
Version control systems (VCS) are tools that help manage changes to source code over time. They enable multiple programmers to collaborate on a project, track changes, and revert to previous versions if necessary. Git and Subversion are widely used version control systems.
Compilers and Interpreters
Compilers and interpreters are tools that translate high-level programming languages into machine code. Compilers translate the entire program at once, while interpreters translate and execute code line by line. Examples of compilers include the GNU Compiler Collection (GCC) and the Microsoft Visual C++ Compiler. Examples of interpreters include the Python interpreter and the Ruby interpreter.
Theoretical Foundations
Automata Theory
Automata theory is the study of abstract machines and the problems they can solve. It provides a theoretical framework for understanding the capabilities and limitations of different computational models. Key concepts in automata theory include finite automata, pushdown automata, and Turing machines.
Computability Theory
Computability theory explores the limits of what can be computed by algorithms. It addresses questions such as whether a given problem is solvable by any algorithm and the resources required to solve it. Important results in computability theory include the Church-Turing thesis and the concept of decidability.
Complexity Theory
Complexity theory studies the resources required to solve computational problems, such as time and space. It classifies problems into complexity classes, such as P, NP, and NP-complete. Complexity theory helps identify problems that are inherently difficult to solve and guides the development of efficient algorithms.
Practical Applications
Software Development
Software development is the process of designing, coding, testing, and maintaining software applications. It involves various stages, including requirements analysis, software design, implementation, testing, and maintenance. Software development methodologies, such as Agile and Waterfall, provide frameworks for managing the development process.
Web Development
Web development involves creating and maintaining websites and web applications. It encompasses both front-end development, which focuses on the user interface and user experience, and back-end development, which deals with server-side logic and database interactions. Technologies used in web development include HTML, CSS, JavaScript, and server-side scripting languages like PHP and Node.js.
Mobile App Development
Mobile app development is the process of creating applications for mobile devices, such as smartphones and tablets. It involves designing user interfaces, implementing functionality, and optimizing performance for mobile platforms. Common tools and frameworks for mobile app development include Android Studio, Xcode, and React Native.
See Also
- Software engineering
- Computer science
- Programming language
- Algorithm
- Data structure
- Integrated development environment