Visitor Pattern
Definition
The Visitor Pattern is a design pattern used in the field of software engineering. It is part of the behavioral pattern group, one of the three design pattern groups identified by the Gang of Four in their foundational book on design patterns in software engineering.
Overview
The Visitor Pattern is a way of separating an algorithm from an object structure it operates on. It allows for new operations to be added without modifying the classes of the elements on which it operates. The pattern is useful when a structure of objects has many different types of elements and we want to perform operations on these elements that depend on their concrete classes.
Structure
The Visitor Pattern consists of the following components:
1. Visitor: This is an interface or an abstract class used to declare the visit operations for all types of visitable classes.
2. ConcreteVisitor: This is a class that implements each operation declared by the Visitor. Each operation implements a fragment of the algorithm needed for the corresponding class of object.
3. Visitable: This is an interface or an abstract class which declares the accept operation. This is the entry point which enables an object to be "visited" by the visitor object.
4. ConcreteVisitable: This is a class that implements the Visitable interface or extends the Visitable class. The visitor object is passed to this object using the accept operation.
Implementation
The implementation of the Visitor Pattern involves creating a Visitor interface with a visit method for each type of element that can be visited. The elements then implement a Visitable interface or abstract class with an accept method that takes a Visitor object as an argument. The Visitor object is then passed to the accept method of the elements to be visited.
Usage
The Visitor Pattern is used when you need to perform an operation on a group of related objects, but want to avoid "polluting" their classes with this operation. It is also useful when the operations to be performed depend on the concrete classes of the objects, and you want to use a mechanism that can handle changes in these classes without having to change the operations.
Advantages and Disadvantages
The Visitor Pattern has several advantages. It allows you to add new operations without modifying the classes of the elements on which they operate. It also makes it easy to add operations that depend on the concrete classes of the elements.
However, the Visitor Pattern also has some disadvantages. It can be difficult to implement in languages that do not support double dispatch. It also makes it hard to add new types of elements, as this would require changing the Visitor interface and all of its implementations.
See Also
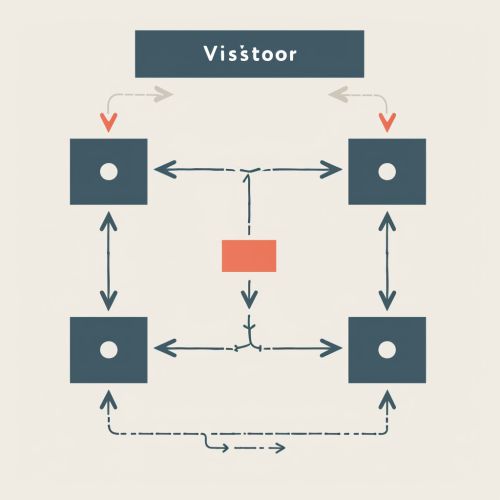
