Thread
Introduction
A thread, in the context of computing, is a sequence of executable instructions that can be managed independently by a scheduler, which is typically a part of the operating system. Threads are a fundamental unit of CPU utilization that form the basis of multithreading, allowing multiple threads to exist within the context of a single process.
Historical Background
The concept of threading emerged as a solution to the limitations of single-threaded processes, which could only execute one instruction sequence at a time. Early computer systems were single-threaded, but as the need for more efficient and responsive systems grew, the concept of threading was developed. The introduction of threading allowed for more efficient use of CPU resources and improved the performance of applications by enabling concurrent execution of tasks.
Types of Threads
Threads can be broadly classified into two categories: user-level threads and kernel-level threads.
User-Level Threads
User-level threads are managed by a user-level library rather than the operating system. The primary advantage of user-level threads is that they can be created and managed without the need for kernel intervention, making them faster and more efficient. However, they have limitations, such as the inability to take full advantage of multiprocessor systems.
Kernel-Level Threads
Kernel-level threads are managed directly by the operating system. These threads can take full advantage of multiprocessor systems, as the kernel can schedule them on different processors. However, they are generally slower to create and manage compared to user-level threads due to the overhead of kernel intervention.
Thread Lifecycle
A thread typically goes through several states during its lifecycle:
New
The thread is created but not yet started.
Runnable
The thread is ready to run and is waiting for CPU time.
Running
The thread is currently executing on the CPU.
Blocked
The thread is waiting for an event, such as I/O operations, to complete.
Terminated
The thread has finished execution.
Thread Synchronization
Thread synchronization is crucial in multithreaded applications to ensure that threads do not interfere with each other. Common synchronization mechanisms include:
Mutexes
A mutex is a mutual exclusion object that prevents multiple threads from accessing a shared resource simultaneously.
Semaphores
A semaphore is a signaling mechanism that controls access to a shared resource by multiple threads.
Monitors
A monitor is a high-level synchronization construct that provides a mechanism for threads to safely access shared resources.
Thread Safety
Thread safety refers to the property of a program or code segment that ensures correct behavior when executed by multiple threads simultaneously. Achieving thread safety often involves using synchronization mechanisms to prevent race conditions and ensure data consistency.
Multithreading Models
There are several models for implementing multithreading in an operating system:
Many-to-One
In the many-to-one model, multiple user-level threads are mapped to a single kernel thread. This model is simple but cannot take advantage of multiprocessor systems.
One-to-One
In the one-to-one model, each user-level thread is mapped to a separate kernel thread. This model provides better concurrency but can be resource-intensive.
Many-to-Many
In the many-to-many model, multiple user-level threads are mapped to an equal or smaller number of kernel threads. This model provides a balance between concurrency and resource usage.
Thread Libraries
Several thread libraries provide APIs for creating and managing threads. Some of the most commonly used thread libraries include:
POSIX Threads (Pthreads)
Pthreads is a POSIX standard for thread creation and synchronization. It is widely used in Unix-like operating systems.
Java Threads
Java provides built-in support for multithreading through the Java Thread class and the java.util.concurrent package.
Windows Threads
The Windows operating system provides the Windows API for creating and managing threads, offering functions such as CreateThread and WaitForSingleObject.
Performance Considerations
While multithreading can significantly improve the performance of applications, it also introduces challenges such as context switching overhead, synchronization overhead, and potential deadlocks. Careful design and optimization are required to maximize the benefits of multithreading while minimizing its drawbacks.
Conclusion
Threads are a fundamental concept in modern computing, enabling concurrent execution of tasks and efficient use of CPU resources. Understanding the different types of threads, their lifecycle, synchronization mechanisms, and performance considerations is essential for developing robust and efficient multithreaded applications.
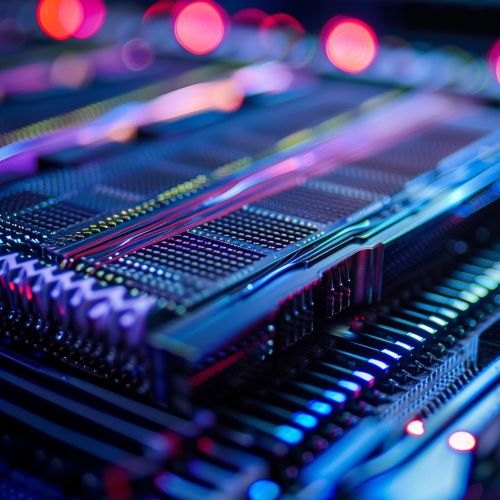
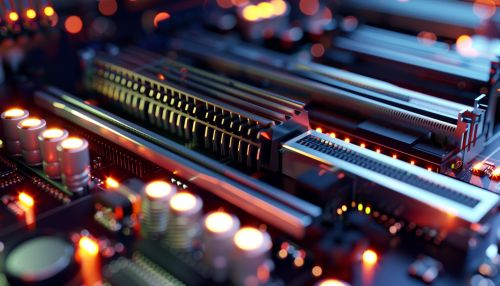