Strategy Pattern
Overview
The Strategy Pattern, also known as the Policy Pattern, is a behavioral design pattern in computer science that enables an algorithm's behavior to be selected at runtime. It is one of the patterns included in the influential book Design Patterns: Elements of Reusable Object-Oriented Software by the Gang of Four.
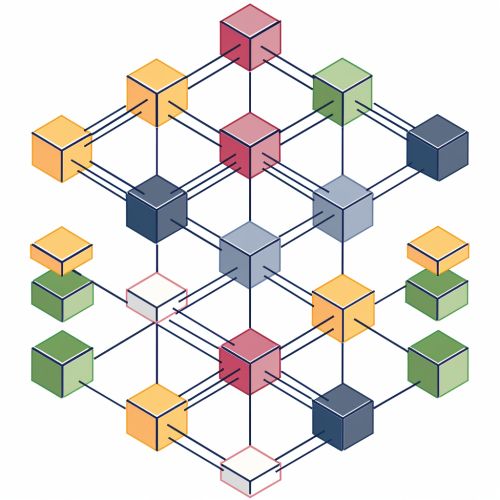
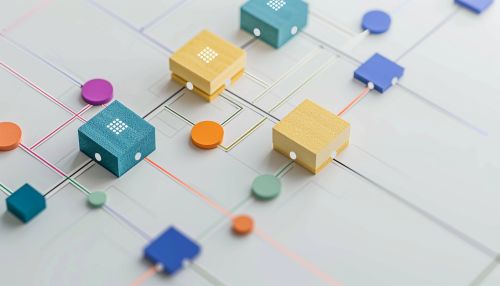
Concept
The Strategy Pattern defines a family of algorithms, encapsulates each one, and makes them interchangeable. This pattern lets the algorithm vary independently from the clients that use it. Strategy Pattern is a type of behavioral pattern, which is concerned with the assignment of responsibilities between objects and how they communicate.
Structure
The Strategy Pattern involves removing an algorithm from its host class and putting it in a separate class. There are three parts to the Strategy Pattern:
1. Context: It maintains a reference to a Strategy object, and is coupled only to the Strategy interface. 2. Strategy: This is an interface common to all supported algorithms. Context uses this interface to call the algorithm defined by a ConcreteStrategy. 3. ConcreteStrategy: It implements the Strategy interface.
Implementation
The Strategy Pattern is implemented by defining a common interface for a set of classes that encapsulate a related set of algorithms or behaviors. An instance of one of these classes (a strategy) is composed within the context object that is to use the strategy. The context object can alter its own behavior by changing its strategy object.
Advantages
The Strategy Pattern has several advantages. It provides a means of defining a family of algorithms or behaviors for a class, without having to define each one within the class itself. It allows the algorithms to be selected at runtime, providing flexibility and extensibility to the software. It also promotes the Open/Closed Principle, as new strategies can be introduced without modifying the existing code.
Disadvantages
However, the Strategy Pattern also has some disadvantages. It can complicate the code by introducing many additional classes. Clients must be aware of the different Strategies to select the appropriate one. It also increases the number of objects in the system, which can increase memory usage and potentially impact performance.
Use Cases
The Strategy Pattern is used when different variations of an algorithm are required. It is also used when these variations need to be selectable at runtime. Examples of the Strategy Pattern in real-world software include the sorting behavior in a database system, the routing behavior in a logistics application, or the compression behavior in a file storage system.