Separate chaining
Separate Chaining
Separate chaining is a method used in computer science to resolve hash table collisions. It is one of the most common techniques employed to handle situations where multiple keys hash to the same index in a hash table. This article delves into the intricacies of separate chaining, exploring its implementation, performance, advantages, and disadvantages.
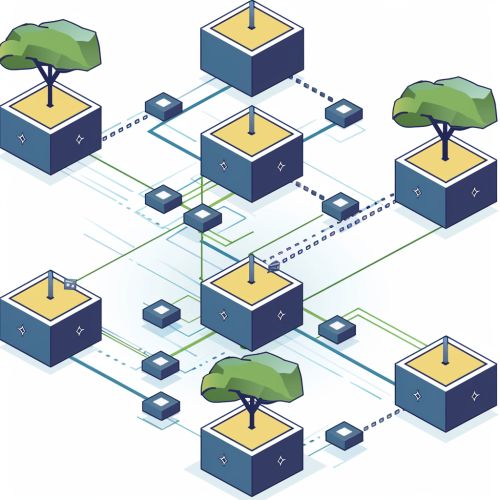
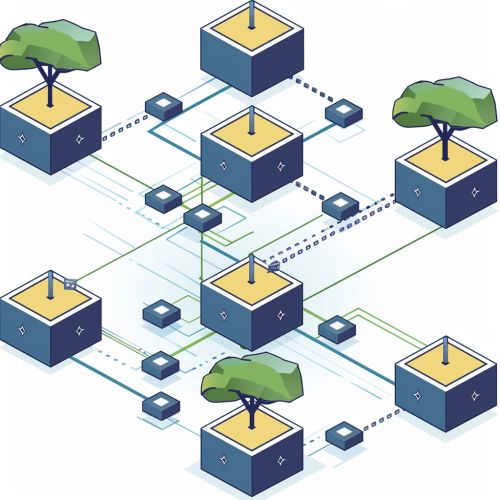
Overview
In a hash table, a hash function maps keys to indices in an array. Ideally, each key maps to a unique index, but in practice, collisions occur when multiple keys hash to the same index. Separate chaining addresses this issue by maintaining a list of all elements that hash to the same index. Each element in the hash table array points to the head of a linked list that contains all the keys that hash to that index.
Implementation
The implementation of separate chaining involves several key components:
- **Hash Function**: A function that maps keys to indices in the hash table.
- **Array of Pointers**: An array where each element points to the head of a linked list.
- **Linked Lists**: Each linked list contains all the keys that hash to the same index.
Hash Function
The choice of the hash function is critical for the performance of the hash table. A good hash function distributes keys uniformly across the array, minimizing the number of collisions. Common hash functions include division method, multiplication method, and universal hashing.
Array of Pointers
The array of pointers is the primary structure of the hash table. Each element in the array points to the head of a linked list. If no keys hash to a particular index, the array element points to `NULL`.
Linked Lists
Each linked list contains nodes, where each node stores a key-value pair and a pointer to the next node. When a new key is inserted, it is added to the beginning of the linked list at the appropriate index.
Performance
The performance of separate chaining depends on several factors, including the quality of the hash function, the load factor, and the average length of the linked lists.
Time Complexity
- **Insertion**: O(1) on average, assuming a good hash function and a low load factor.
- **Search**: O(1) on average, but O(n) in the worst case if all keys hash to the same index.
- **Deletion**: O(1) on average, similar to insertion and search.
Load Factor
The load factor (λ) is defined as the ratio of the number of keys (n) to the number of slots in the hash table (m): λ = n/m. A lower load factor reduces the average length of the linked lists, improving performance.
Advantages
- **Simplicity**: Separate chaining is straightforward to implement.
- **Flexibility**: The hash table can handle an arbitrary number of keys without resizing.
- **Performance**: With a good hash function, separate chaining provides constant-time operations on average.
Disadvantages
- **Memory Overhead**: Separate chaining requires additional memory for the pointers in the linked lists.
- **Cache Performance**: The linked lists may lead to poor cache performance due to non-contiguous memory access.
- **Worst-Case Performance**: In the worst case, where all keys hash to the same index, the performance degrades to O(n).
Variations
Several variations of separate chaining exist to address its limitations:
- **Self-Adjusting Lists**: Techniques like move-to-front heuristic can improve the performance of search operations.
- **Balanced Trees**: Using balanced trees instead of linked lists can improve the worst-case performance.
- **Dynamic Resizing**: Dynamically resizing the hash table and rehashing the keys can maintain a low load factor.
Applications
Separate chaining is widely used in various applications, including:
- **Symbol Tables**: In compilers and interpreters, symbol tables use hash tables with separate chaining to manage identifiers.
- **Databases**: Hash tables with separate chaining are used in databases for indexing and quick lookups.
- **Caches**: Caching systems use hash tables to store and retrieve data efficiently.