Semaphore
Introduction
A semaphore is a synchronization mechanism used in computer science to control access to a common resource by multiple processes and to prevent critical section problems in concurrent programming. Originating from the Greek word "sēma," meaning sign or signal, semaphores are essential in managing process synchronization and ensuring that multiple processes do not simultaneously access a shared resource, which could lead to data inconsistency or corruption.
Types of Semaphores
Semaphores can be broadly classified into two types: binary semaphores and counting semaphores.
Binary Semaphores
Binary semaphores, also known as mutexes (mutual exclusions), can only take two values: 0 and 1. They are used to manage access to a single resource. When a process acquires the semaphore, its value changes to 0, indicating that the resource is in use. When the process releases the semaphore, its value changes back to 1, indicating that the resource is available.
Counting Semaphores
Counting semaphores can take any non-negative integer value and are used to manage access to a resource pool with multiple instances. The semaphore value represents the number of available resources. When a process acquires a resource, the semaphore value is decremented. When a process releases a resource, the semaphore value is incremented.
Semaphore Operations
Semaphores support two primary atomic operations: wait (P) and signal (V).
Wait (P) Operation
The wait operation, also known as P (from the Dutch word "proberen," meaning to test), decrements the semaphore value. If the value is greater than zero, the process continues execution. If the value is zero, the process is blocked until the semaphore value becomes greater than zero.
Signal (V) Operation
The signal operation, also known as V (from the Dutch word "verhogen," meaning to increment), increments the semaphore value. If there are any processes blocked on the semaphore, one of them is unblocked and allowed to proceed.
Implementation of Semaphores
Semaphores can be implemented in various ways, including hardware support, operating system support, and software libraries.
Hardware Support
Some computer architectures provide hardware support for atomic semaphore operations, such as test-and-set or compare-and-swap instructions. These instructions ensure that semaphore operations are performed atomically, preventing race conditions.
Operating System Support
Most modern operating systems provide built-in support for semaphores as part of their process synchronization primitives. For example, POSIX-compliant operating systems offer POSIX semaphores, which can be used in both user-space and kernel-space applications.
Software Libraries
Several programming languages and libraries provide semaphore implementations. For example, the Java programming language includes the `java.util.concurrent.Semaphore` class, and the Python programming language offers the `threading.Semaphore` class.
Use Cases of Semaphores
Semaphores are used in various scenarios to ensure proper synchronization and resource management.
Mutual Exclusion
Semaphores are commonly used to enforce mutual exclusion, ensuring that only one process accesses a critical section at a time. This prevents race conditions and ensures data consistency.
Producer-Consumer Problem
In the producer-consumer problem, semaphores are used to synchronize access to a shared buffer. The producer adds items to the buffer, and the consumer removes items from the buffer. Semaphores ensure that the producer does not add items to a full buffer and the consumer does not remove items from an empty buffer.
Reader-Writer Problem
The reader-writer problem involves synchronizing access to a shared resource, such as a database, where multiple readers can read simultaneously, but writers require exclusive access. Semaphores are used to manage the access, ensuring that readers and writers do not interfere with each other.
Advantages and Disadvantages of Semaphores
Semaphores offer several advantages and disadvantages in process synchronization.
Advantages
- **Simplicity**: Semaphores provide a simple and effective mechanism for process synchronization.
- **Flexibility**: Semaphores can be used to solve various synchronization problems, such as mutual exclusion, producer-consumer, and reader-writer problems.
- **Efficiency**: Semaphore operations are typically efficient and can be implemented with minimal overhead.
Disadvantages
- **Complexity**: Proper use of semaphores requires careful design to avoid issues such as deadlocks and priority inversion.
- **Scalability**: Semaphores may not scale well in systems with a large number of processes or threads, leading to contention and performance degradation.
- **Error-Prone**: Incorrect use of semaphores can lead to subtle and hard-to-debug synchronization issues.
Semaphore in Operating Systems
Operating systems use semaphores extensively to manage process synchronization and resource allocation.
Process Synchronization
Semaphores are used to synchronize processes and threads, ensuring that they do not interfere with each other while accessing shared resources. This is critical in multi-threaded and multi-process environments.
Resource Allocation
Operating systems use semaphores to manage resource allocation, such as memory, CPU time, and I/O devices. Semaphores ensure that resources are allocated fairly and efficiently, preventing resource starvation and contention.
Semaphore in Distributed Systems
In distributed systems, semaphores are used to synchronize processes running on different machines. This requires additional mechanisms to ensure that semaphore operations are performed atomically across the network.
Distributed Mutual Exclusion
Semaphores can be used to implement distributed mutual exclusion, ensuring that only one process in the distributed system accesses a critical section at a time. This is essential for maintaining data consistency and preventing race conditions in distributed applications.
Distributed Resource Allocation
Semaphores are used to manage resource allocation in distributed systems, ensuring that resources are allocated fairly and efficiently across multiple machines. This is critical for maintaining system performance and preventing resource contention.
Semaphore in Real-Time Systems
In real-time systems, semaphores are used to ensure that tasks meet their deadlines and execute in a predictable manner.
Task Synchronization
Semaphores are used to synchronize tasks, ensuring that they execute in the correct order and do not interfere with each other. This is critical for maintaining the timing constraints of real-time systems.
Resource Management
Semaphores are used to manage resources in real-time systems, ensuring that tasks have access to the resources they need to execute on time. This is essential for maintaining the predictability and reliability of real-time systems.
Advanced Topics in Semaphores
Several advanced topics in semaphores are relevant for expert-level understanding.
Priority Inversion
Priority inversion occurs when a lower-priority process holds a semaphore needed by a higher-priority process, causing the higher-priority process to be blocked. This can lead to performance degradation and missed deadlines in real-time systems. Solutions to priority inversion include priority inheritance and priority ceiling protocols.
Deadlocks
Deadlocks occur when two or more processes are blocked, each waiting for a semaphore held by another process. This can lead to system hangs and resource starvation. Deadlock prevention and detection techniques are used to mitigate this issue.
Semaphore Algorithms
Several algorithms have been developed to optimize semaphore operations, such as the bakery algorithm and the Lamport's algorithm. These algorithms ensure that semaphore operations are performed efficiently and fairly.
Conclusion
Semaphores are a fundamental synchronization mechanism in computer science, used to manage access to shared resources and prevent critical section problems. They are used in various scenarios, including mutual exclusion, producer-consumer, and reader-writer problems. While semaphores offer several advantages, they also have limitations, such as complexity and scalability issues. Understanding the proper use of semaphores is essential for designing efficient and reliable concurrent systems.
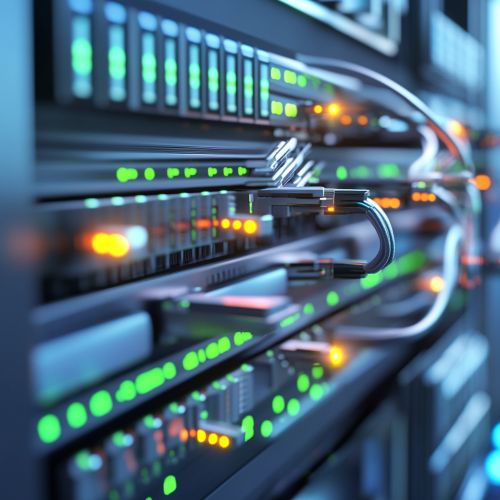
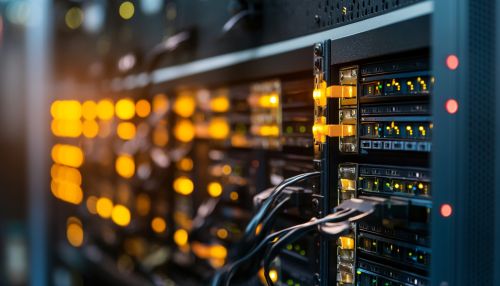