Python Implementation of Modbus RTU
Overview
Modbus is a communication protocol developed in 1979 for use with programmable logic controllers (PLCs). Modbus RTU, a variant of the Modbus protocol, is widely used in industrial control systems and has been implemented in many different hardware and software platforms. This article discusses the implementation of Modbus RTU in Python, a high-level, interpreted programming language that is popular for its simplicity and versatility.
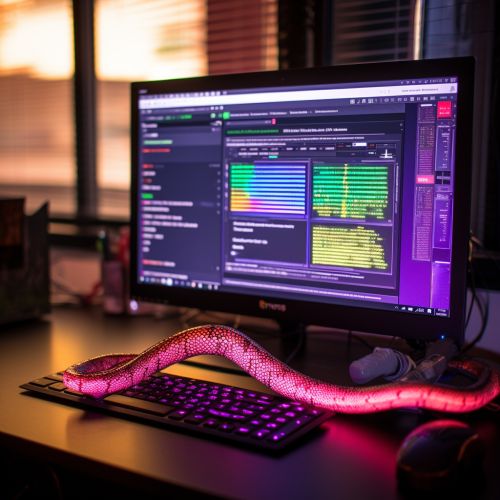
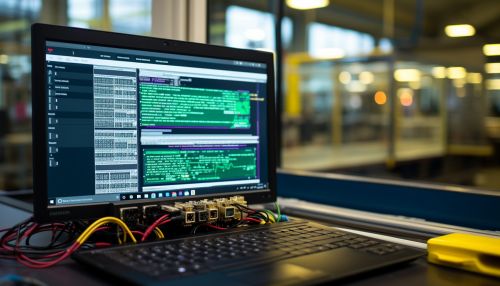
Modbus RTU Protocol
Modbus RTU (Remote Terminal Unit) is a binary protocol that uses a compact, binary representation of the data for protocol communication. It is typically used over RS-485 and RS-232 interfaces for communication between devices. The protocol is simple and robust, making it easy to use for real-time applications.
The Modbus RTU message consists of the address of the slave device, a function code defining the requested action, any data to be sent, and an error-checking field. The data and the error-checking field are packed as binary data.
Python and Modbus RTU
Python is a popular language for implementing Modbus RTU due to its simplicity and the availability of libraries that simplify the process. The most commonly used library for Modbus RTU in Python is pymodbus, a full-featured and mature library that supports both Modbus RTU and Modbus TCP/IP.
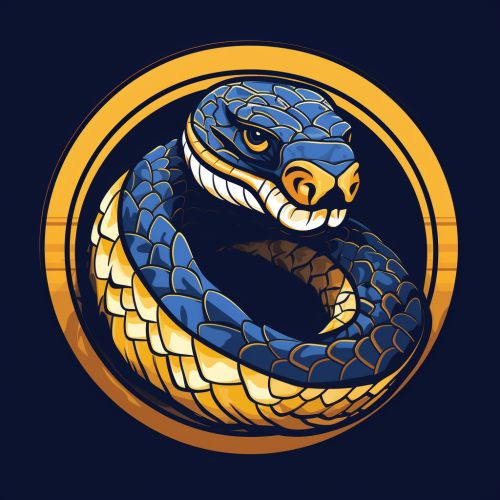
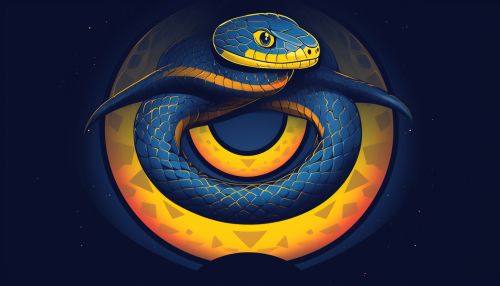
Implementing Modbus RTU in Python
To implement Modbus RTU in Python, you will need to install the pymodbus library. This can be done using pip, a package manager for Python.
Once the library is installed, you can create a Modbus client or server using the pymodbus library. The client is used to send requests to the server, which responds to the requests.
Creating a Modbus RTU Client
To create a Modbus RTU client, you will need to import the ModbusSerialClient class from the pymodbus.client.sync module. You can then create an instance of the ModbusSerialClient class, specifying the serial port and other parameters.
Creating a Modbus RTU Server
Creating a Modbus RTU server is similar to creating a client. You will need to import the ModbusServer and ModbusSequentialDataBlock classes from the pymodbus.server.sync module. You can then create an instance of the ModbusServer class, specifying the serial port and other parameters.
Example Code
The following is an example of a simple Modbus RTU client and server in Python using the pymodbus library.
Modbus RTU Client
```python from pymodbus.client.sync import ModbusSerialClient
client = ModbusSerialClient('rtu', port='/dev/ttyUSB0', baudrate=9600)
client.connect()
result = client.read_coils(1, 10)
print(result.bits)
client.close() ```
Modbus RTU Server
```python from pymodbus.server.sync import ModbusServer, ModbusSequentialDataBlock
server = ModbusServer('/dev/ttyUSB0', 9600, ModbusSequentialDataBlock(0, [0]*100))
server.start() ```
Conclusion
Python's simplicity and the availability of libraries like pymodbus make it an excellent choice for implementing Modbus RTU. Whether you're creating a client to send requests or a server to respond to them, Python and pymodbus provide all the tools you need.
See Also
-
Processing started
-
Rate limit check
-
Title check
-
Suitability check
-
Text generation (step 2 of 2)
-
Images pre-generation (0 of 6 images are ready)
-
Images generation (4 of 6 images are ready)
-
Publication