Mean Shift
Mean Shift
Mean Shift is a non-parametric iterative algorithm primarily used for clustering and mode-seeking in data analysis. This technique is particularly useful in the field of computer vision and image processing, as well as in other domains requiring pattern recognition and data segmentation. The algorithm works by shifting data points towards the mode (i.e., the highest density of data points) in a feature space, thus identifying clusters without requiring prior knowledge of the number of clusters.
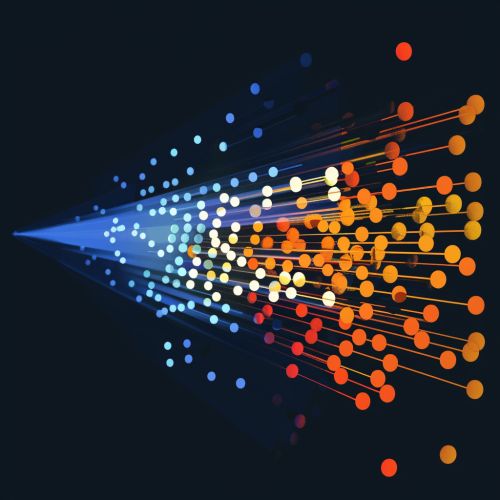
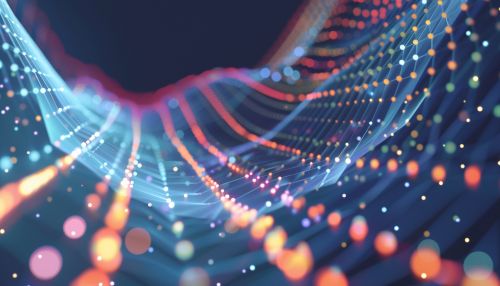
Background and History
Mean Shift was first introduced by Fukunaga and Hostetler in 1975. The algorithm gained popularity due to its simplicity and effectiveness in various applications. Unlike traditional clustering methods such as K-means, which require the number of clusters to be specified in advance, Mean Shift dynamically finds the number of clusters by locating the modes in the data distribution.
Algorithm Description
The Mean Shift algorithm operates by iteratively shifting each data point towards the mean of the points within a given neighborhood. This process continues until convergence, where the points no longer move significantly. The steps of the algorithm are as follows:
1. **Initialization**: Start with a set of data points in the feature space. 2. **Kernel Density Estimation**: For each data point, compute the mean of the points within a specified radius (bandwidth). 3. **Shift**: Move the data point towards the computed mean. 4. **Convergence**: Repeat steps 2 and 3 until the data points converge to the modes of the distribution.
The choice of the kernel and bandwidth is crucial for the performance of the algorithm. Commonly used kernels include the Gaussian kernel and the Epanechnikov kernel.
Mathematical Formulation
The Mean Shift vector is defined as:
\[ m(x) = \frac{\sum_{i=1}^{n} K(x_i - x)x_i}{\sum_{i=1}^{n} K(x_i - x)} - x \]
where \( x \) is the current data point, \( x_i \) are the neighboring points, \( K \) is the kernel function, and \( n \) is the number of points within the bandwidth.
The update rule for the data point is:
\[ x \leftarrow x + m(x) \]
This process is repeated until \( m(x) \) is smaller than a predefined threshold, indicating convergence.
Applications
Mean Shift has been successfully applied in various fields, including:
- **Computer Vision**: Object tracking, image segmentation, and feature space analysis.
- **Image Processing**: Denoising, edge detection, and color space analysis.
- **Pattern Recognition**: Clustering, mode detection, and anomaly detection.
- **Robotics**: Path planning and obstacle avoidance.
In computer vision, Mean Shift is often used for image segmentation by clustering pixels based on their color and spatial information. This allows for the identification of distinct objects and regions within an image.
Advantages and Limitations
- Advantages**:
- Non-parametric: Does not require prior knowledge of the number of clusters.
- Robust to outliers: The algorithm can handle noisy data effectively.
- Versatile: Applicable to various types of data and feature spaces.
- Limitations**:
- Computationally Intensive: The iterative nature of the algorithm can be slow for large datasets.
- Bandwidth Selection: The choice of bandwidth significantly affects the results and may require tuning.
- Convergence Issues: The algorithm may converge to local modes, leading to suboptimal clustering.
Variants and Extensions
Several variants and extensions of the Mean Shift algorithm have been proposed to address its limitations and enhance its performance:
- **Adaptive Mean Shift**: Adjusts the bandwidth dynamically based on the local density of data points.
- **Mean Shift with Annealing**: Incorporates an annealing process to escape local modes and improve convergence.
- **Hierarchical Mean Shift**: Builds a hierarchy of clusters to provide multi-scale analysis of the data.
These variants aim to improve the robustness, efficiency, and scalability of the Mean Shift algorithm, making it suitable for a wider range of applications.
Implementation
Mean Shift can be implemented in various programming languages, including Python, MATLAB, and C++. Popular libraries such as scikit-learn provide built-in functions for Mean Shift clustering, making it accessible to practitioners and researchers.
A basic implementation in Python using scikit-learn is as follows:
```python from sklearn.cluster import MeanShift import numpy as np
- Sample data
X = np.array([[1, 2], [2, 3], [3, 4], [8, 7], [8, 8], [25, 80]])
- Mean Shift clustering
ms = MeanShift() ms.fit(X) labels = ms.labels_ cluster_centers = ms.cluster_centers_
print("Labels:", labels) print("Cluster Centers:", cluster_centers) ```
This code demonstrates the simplicity of applying Mean Shift clustering to a set of data points.