Libc
Overview
The C standard library, commonly referred to as "libc," is a collection of standardized functions and macros that provide essential services for C programs. These services include input/output operations, memory management, string manipulation, and mathematical computations. The library is fundamental to the C programming language and is implemented in various forms across different operating systems and compilers.
History
The origins of libc can be traced back to the early days of the C programming language and the UNIX operating system. Dennis Ritchie and Ken Thompson developed the initial version of the C library as part of the UNIX operating system at Bell Labs in the early 1970s. The library has since evolved and expanded, becoming a cornerstone of modern computing.
Structure and Components
Libc is divided into several key components, each providing a specific set of functionalities:
Standard I/O
The standard input/output library provides functions for reading from and writing to files, as well as standard input and output streams. Key functions include `fopen`, `fclose`, `fread`, `fwrite`, `fprintf`, and `fscanf`.
String Handling
String handling functions allow for manipulation of C-style strings, which are null-terminated character arrays. Important functions include `strcpy`, `strncpy`, `strcat`, `strncat`, `strcmp`, `strncmp`, `strlen`, and `strchr`.
Memory Management
Memory management functions provide dynamic allocation and deallocation of memory. The primary functions are `malloc`, `calloc`, `realloc`, and `free`.
Mathematical Functions
The mathematical functions in libc provide a range of operations, from basic arithmetic to complex trigonometric and logarithmic calculations. Functions include `sin`, `cos`, `tan`, `exp`, `log`, `sqrt`, and `pow`.
Utility Functions
Libc also includes various utility functions for tasks such as sorting and searching. Functions like `qsort` and `bsearch` are commonly used for these purposes.
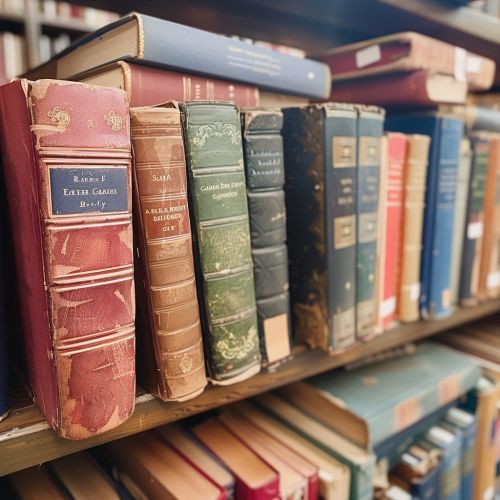
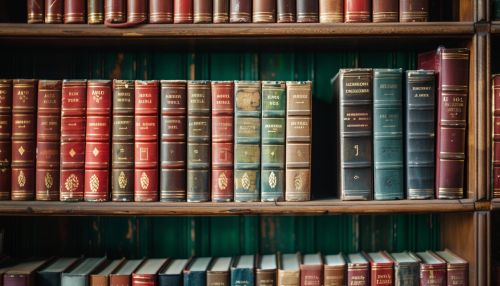
Implementation Variants
There are several implementations of libc, each tailored to specific operating systems and environments. Some of the most notable implementations include:
GNU C Library (glibc)
The GNU C Library, or glibc, is the most widely used implementation of libc on Linux systems. It is maintained by the GNU Project and provides extensive functionality, including support for internationalization and threading.
musl
Musl is a lightweight, fast, and simple implementation of libc designed for static linking and embedded systems. It aims to be standards-compliant and is used in various Linux distributions, such as Alpine Linux.
Bionic
Bionic is the libc implementation used in the Android operating system. It is designed to be lightweight and efficient, with specific optimizations for mobile devices.
uClibc
The uClibc (micro C library) is a smaller, more efficient version of libc intended for use in embedded systems. It provides a subset of the functionality of glibc, making it suitable for resource-constrained environments.
Standards Compliance
Libc is designed to comply with various standards, ensuring portability and interoperability across different systems. Key standards include:
ANSI C
The American National Standards Institute (ANSI) C standard, also known as C89 or C90, defines the core set of functions and macros that make up the C standard library.
POSIX
The Portable Operating System Interface (POSIX) standard defines a set of APIs for compatibility between UNIX-like operating systems. Libc implementations often include POSIX-compliant functions to ensure compatibility with a wide range of systems.
ISO C
The International Organization for Standardization (ISO) C standard, also known as C99 and later revisions such as C11 and C18, extends the ANSI C standard with additional features and improvements.
Security Considerations
Libc functions are critical to the operation of many programs, and their misuse can lead to security vulnerabilities. Common issues include buffer overflows, memory leaks, and improper handling of input data. Developers must exercise caution when using libc functions and follow best practices for secure coding.
Performance Optimization
Optimizing the performance of libc functions is crucial for high-performance applications. Techniques such as inline assembly, algorithmic improvements, and hardware-specific optimizations are often employed to enhance the efficiency of libc functions.
Future Developments
The development of libc continues to evolve, with ongoing efforts to improve performance, security, and standards compliance. Future versions of libc may include support for new programming paradigms, enhanced parallelism, and better integration with modern hardware architectures.