Interpreter Pattern
Overview
The Interpreter Pattern is a type of behavioral design pattern that specifies how to evaluate sentences in a language. This pattern involves implementing a language interpreter for a defined grammar, including a representation for the grammar along with an interpreter that uses the representation to interpret sentences in the language. The key idea in this pattern is to define a domain language (also known as a DSL) that can solve a particular type of problem.
Design Principles
The Interpreter Pattern is based on the principle of separation of concerns, which means different aspects of the software should be dealt with by distinct components. This pattern separates the grammar of a language from its interpretation. It encapsulates the complexities of parsing and interpreting a language into separate classes, thus making it easier to understand and maintain.
Structure
The Interpreter Pattern consists of the following components:
- AbstractExpression: This is an abstract class that declares an abstract Interpret operation that all nodes (terminal and nonterminal) in the AST must implement.
- TerminalExpression: This is a class that implements the Interpret operation for terminal expressions in the grammar. Terminal expressions are the leaf nodes of the AST.
- NonterminalExpression: This is a class that implements the Interpret operation for nonterminal expressions in the grammar. Nonterminal expressions are the internal nodes of the AST.
- Context: This class contains global information that is used and updated during the interpretation process.
- Client: This class builds the AST and invokes the Interpret operation.
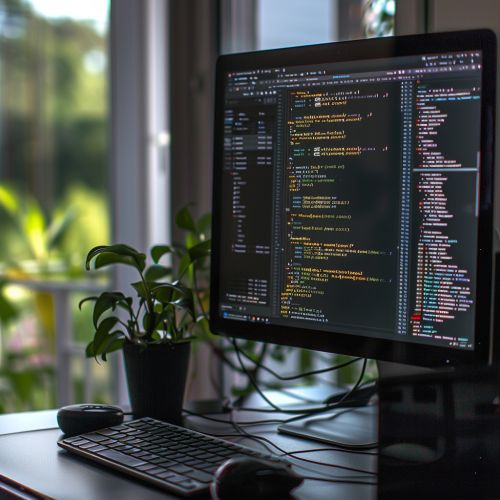
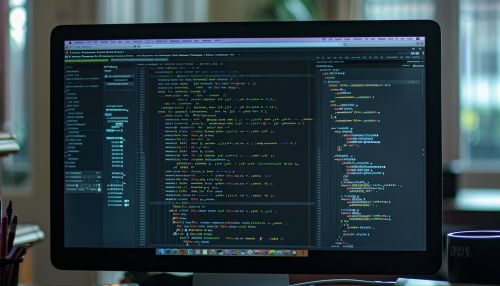
Usage
The Interpreter Pattern is used when a language needs to be interpreted and the grammar of the language can be represented as a simple abstract syntax tree (AST). It is commonly used in compilers, interpreters, and IDEs. However, it should be noted that this pattern can lead to a large number of classes for complex grammars and thus should be used judiciously.
Benefits and Drawbacks
The Interpreter Pattern has several benefits:
- It provides a way to include language operations in the grammar. The pattern uses an abstract syntax tree, which makes it easy to include operations on the language constructs.
- It allows extending the language. Since the grammar is represented as classes, new ways to interpret expressions can be easily added by creating new operations on these classes.
However, the Interpreter Pattern also has some drawbacks:
- It can lead to complex class hierarchies for complex grammars.
- It can be hard to maintain and modify, especially when the grammar changes.
Examples
One common example of the Interpreter Pattern is in regular expression engines. A regular expression is a type of language, and a regular expression engine is an interpreter for that language. The engine constructs an abstract syntax tree from the regular expression, and then interprets this tree to perform pattern-matching operations.
Another example is in the implementation of compilers and interpreters for programming languages. The compiler or interpreter first constructs an abstract syntax tree from the source code, and then interprets this tree to execute the program.
See Also
Design Patterns in Software Engineering Behavioral Design Patterns Abstract Syntax Tree in Compiler Design