Event-Driven Programming
Introduction
Event-driven programming is a programming paradigm that primarily uses events to drive the program execution. An event can be defined as a significant occurrence or change in state within the system or application. This paradigm is widely used in graphical user interfaces, real-time systems, and server applications.
Concept
In event-driven programming, the flow of the program is determined by events such as user actions, sensor outputs, or messages from other programs. Events are monitored by event listeners. When an event occurs, an event handler is invoked which executes a callback function.
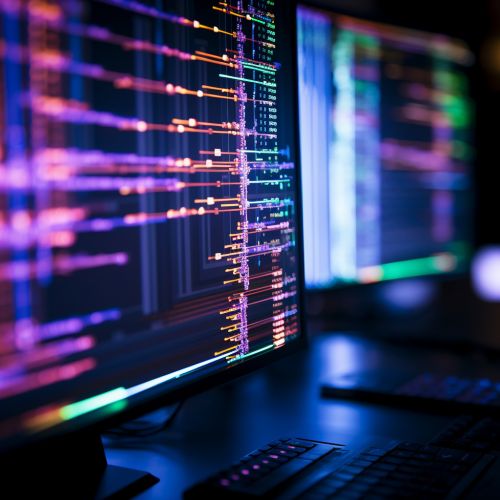
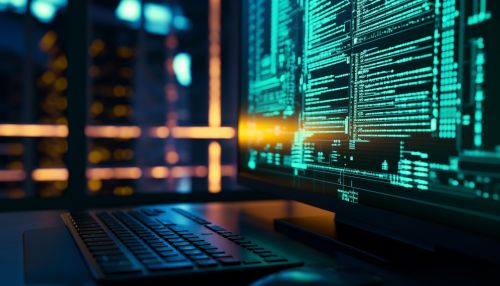
Characteristics
Event-driven programming has several key characteristics:
- Event Listeners: These are procedures or functions in a program that wait for an event to occur.
- Event Handlers: Once the event is detected, event handlers are triggered to respond to the event.
- Event Loop: In an event-driven application, an event loop continuously listens for events and triggers the appropriate event handler.
- Callback Functions: These are functions that are passed as arguments to other functions to be invoked or "called back" at the appropriate time.
Advantages
Event-driven programming offers several advantages:
- Responsiveness: Since events are handled as soon as they are raised, event-driven programs are often more responsive to real-time user input than other types of programs.
- Simplicity: The event-driven model simplifies programming for environments that are naturally event-driven, like user interfaces.
- Resource Usage: Event-driven programming can be more efficient than other paradigms in situations where the program is largely waiting for external events to happen.
Disadvantages
However, event-driven programming also has some disadvantages:
- Complexity: In large applications, managing and debugging the flow of events can become complex.
- Concurrency: As event-driven programming is inherently concurrent, it can lead to issues such as race conditions if not managed properly.
Event-Driven Programming in Different Languages
Event-driven programming is supported in many programming languages through their standard libraries or third-party libraries. Here are a few examples:
- JavaScript: JavaScript is inherently event-driven, with events like onclick or onload driving the execution of web pages.
- Python: Python supports event-driven programming through libraries like Tkinter for GUI development and asyncio for asynchronous I/O.
- Java: Java has built-in support for event-driven programming in its Abstract Window Toolkit (AWT) and Swing libraries.