Binary tree
Definition
A binary tree is a type of data structure that has at most two children for each parent node. The children are usually distinguished as "left" and "right". Nodes with children are parent nodes, or simply parents. While binary trees are used in many areas of computer science, they are most commonly used to implement binary search trees and binary heaps.
Properties
Binary trees have several properties that are useful in algorithms and data structures. The height of a binary tree is the maximum number of edges from the root node to any leaf node. The maximum number of nodes in a binary tree of height 'h' is 2^h - 1. Conversely, the minimum possible height of a binary tree with 'n' nodes is log(n+1). These properties are important in many algorithms that use binary trees.
Types of Binary Trees
There are several types of binary trees, each with different properties and uses.
Full Binary Tree
A full binary tree (also known as a proper or plane binary tree) is a binary tree in which every node has either 0 or 2 children. Full binary trees are used in some very efficient search algorithms.
Complete Binary Tree
A complete binary tree is a binary tree in which every level, except possibly the last, is completely filled, and all nodes are as far left as possible. Complete binary trees are used to implement binary heaps.
Perfect Binary Tree
A perfect binary tree is a binary tree in which all interior nodes have two children and all leaves have the same depth or same level. Perfect binary trees are used in some specialized algorithms, such as in the implementation of deterministic sorting algorithms.
Balanced Binary Tree
A balanced binary tree is a binary tree structure in which the left and right subtrees of every node differ in height by no more than 1. Balanced binary trees are used in algorithms that need to maintain data in a sorted order, such as AVL trees.
Algorithms
Binary trees are used in many algorithms. Some of the most common algorithms that use binary trees include:
Tree Traversal
Tree traversal is a common operation performed on binary trees. There are three common types of binary tree traversal: pre-order, in-order, and post-order.
Pre-order Traversal
In a pre-order traversal, the root node is visited first, then the left subtree, and finally the right subtree.
In-order Traversal
In an in-order traversal, the left subtree is visited first, then the root node, and finally the right subtree. In-order traversal of a binary search tree always gives nodes in non-decreasing order.
Post-order Traversal
In a post-order traversal, the left subtree is visited first, then the right subtree, and finally the root node.
Binary Search Tree
A binary search tree is a type of binary tree used in computer science for efficient searching. It allows for fast lookup, addition, and removal of items.
Applications
Binary trees have many practical applications. They are used in many areas of computer science, including operating systems, graphics, database systems, and computer networking. Binary trees are also used in numerical computation, for example in the implementation of binary space partitioning.
See Also
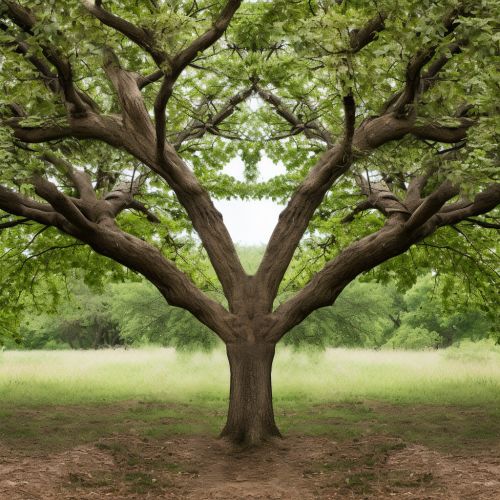
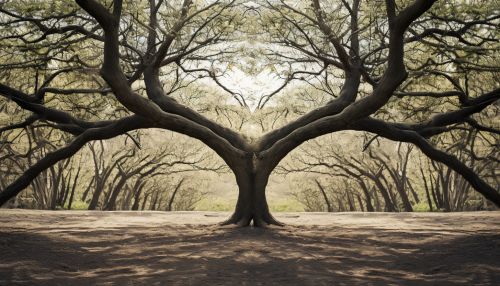