Bellman-Ford Algorithm
Introduction
The Bellman-Ford algorithm is a graph search algorithm that computes shortest paths from a single source vertex to all other vertices in a weighted graph. It is more versatile than Dijkstra's algorithm, as it can handle graphs in which some of the edge weights are negative.
History
The Bellman-Ford algorithm was developed by Richard Bellman and Lester Ford Jr. in 1958. The algorithm was later improved by Edward F. Moore in 1959. The algorithm is based on the principle of relaxation, in which an approximation to the correct distance is gradually replaced by more accurate values until eventually reaching the optimum solution.
Algorithm Description
The Bellman-Ford algorithm operates by iteratively relaxing the edges of the graph. In each iteration, the algorithm traverses the graph and attempts to minimize the cost of each vertex by checking if the cost of reaching that vertex via its neighbors is less than its current cost. If so, the cost is updated to the lower value.
Pseudocode
The pseudocode for the Bellman-Ford algorithm is as follows:
``` function BellmanFord(graph, source):
distance[source] = 0 for each vertex v in graph: if v ≠ source: distance[v] = ∞ predecessor[v] = undefined for i from 1 to size(vertices)-1: for each edge (u, v) with weight w in edges: if distance[u] + w < distance[v]: distance[v] = distance[u] + w predecessor[v] = u for each edge (u, v) with weight w in edges: if distance[u] + w < distance[v]: error "Graph contains a negative-weight cycle" return distance[], predecessor[]
```
Time Complexity
The time complexity of the Bellman-Ford algorithm is O(|V|.|E|), where |V| is the number of vertices and |E| is the number of edges in the graph. This makes it less efficient than Dijkstra's algorithm for sparse graphs, but more versatile, as it can handle graphs with negative edge weights.
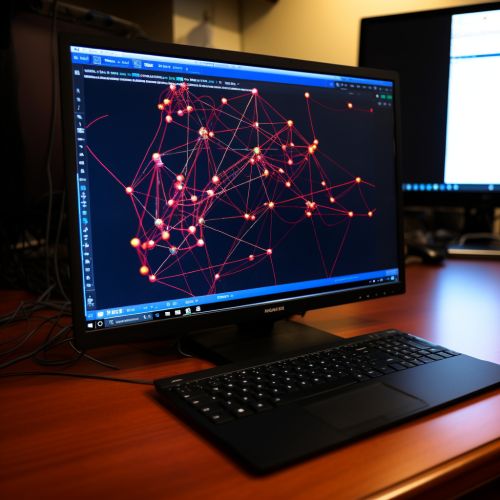
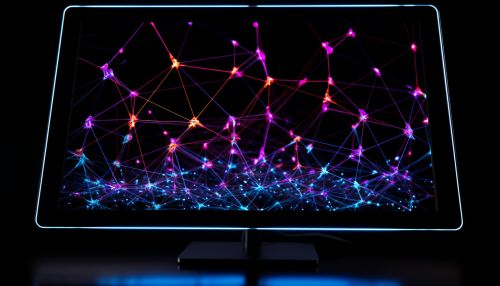
Applications
The Bellman-Ford algorithm has several applications in network routing protocols, notably the Routing Information Protocol (RIP). RIP is an Interior Gateway Protocol (IGP) used to distribute routing information within networks, such as local area networks (LANs) and wide area networks (WANs).
Limitations
While the Bellman-Ford algorithm is versatile and can handle negative edge weights, it is not without its limitations. The algorithm does not work when there are negative cycles in the graph, as the cost to reach some vertices could be reduced indefinitely. Another limitation is its time complexity, which is higher than that of some other shortest path algorithms, such as Dijkstra's algorithm.