Quicksort: Difference between revisions
(Created page with "== Introduction == Quicksort is a highly efficient and widely used sorting algorithm in computer science. Developed by British computer scientist Tony Hoare in 1959, Quicksort is a comparison sort and, in most practical scenarios, performs better than other O(n log n) algorithms such as merge sort and heapsort. The algorithm employs a divide-and-conquer strategy to sort elements, making it a fundamental topic in the study of algorithms and data structures. == Algorithm...") |
No edit summary |
||
Line 35: | Line 35: | ||
The Hoare partition scheme, named after the algorithm's inventor, uses two indices that start at the ends of the array being partitioned, then move toward each other until they detect an inversion. This scheme is generally more efficient than the Lomuto scheme but is slightly more complex to implement. | The Hoare partition scheme, named after the algorithm's inventor, uses two indices that start at the ends of the array being partitioned, then move toward each other until they detect an inversion. This scheme is generally more efficient than the Lomuto scheme but is slightly more complex to implement. | ||
[[Image:Detail-98237.jpg|thumb|center|Illustration of the partitioning process in Quicksort.|class=only_on_mobile]] | |||
[[Image:Detail-98238.jpg|thumb|center|Illustration of the partitioning process in Quicksort.|class=only_on_desktop]] | |||
== Performance Analysis == | == Performance Analysis == |
Latest revision as of 20:37, 8 October 2024
Introduction
Quicksort is a highly efficient and widely used sorting algorithm in computer science. Developed by British computer scientist Tony Hoare in 1959, Quicksort is a comparison sort and, in most practical scenarios, performs better than other O(n log n) algorithms such as merge sort and heapsort. The algorithm employs a divide-and-conquer strategy to sort elements, making it a fundamental topic in the study of algorithms and data structures.
Algorithm Description
Quicksort operates by selecting a 'pivot' element from the array and partitioning the other elements into two sub-arrays, according to whether they are less than or greater than the pivot. The sub-arrays are then sorted recursively. This process is repeated until the base case of an empty or single-element array is reached, which is inherently sorted.
Pseudocode
The following pseudocode outlines the basic steps of the Quicksort algorithm:
``` function quicksort(array, low, high) is
if low < high then pivot_index := partition(array, low, high) quicksort(array, low, pivot_index - 1) quicksort(array, pivot_index + 1, high)
function partition(array, low, high) is
pivot := array[high] i := low - 1 for j := low to high - 1 do if array[j] <= pivot then i := i + 1 swap array[i] with array[j] swap array[i + 1] with array[high] return i + 1
```
Partitioning Schemes
The efficiency of Quicksort largely depends on the partitioning scheme used. Several partitioning schemes have been developed to optimize the algorithm's performance under different conditions.
Lomuto Partition Scheme
The Lomuto partition scheme, as shown in the pseudocode above, uses the last element as the pivot. It is simple to implement but can be inefficient for certain input sequences, especially those that are already sorted or nearly sorted.
Hoare Partition Scheme
The Hoare partition scheme, named after the algorithm's inventor, uses two indices that start at the ends of the array being partitioned, then move toward each other until they detect an inversion. This scheme is generally more efficient than the Lomuto scheme but is slightly more complex to implement.
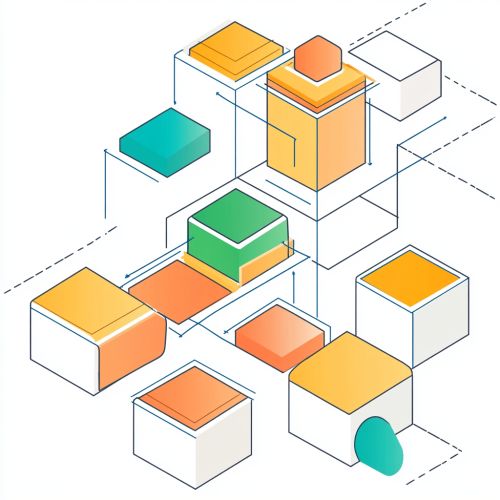

Performance Analysis
Quicksort's performance is influenced by the choice of pivot and the partitioning scheme. Its average-case time complexity is O(n log n), but its worst-case time complexity is O(n^2), which occurs when the smallest or largest element is always chosen as the pivot.
Best Case
The best-case scenario for Quicksort occurs when the pivot element divides the array into two nearly equal halves. This results in a time complexity of O(n log n).
Average Case
In the average case, Quicksort also has a time complexity of O(n log n). This is because the partitioning process is expected to produce sub-arrays of roughly equal size, leading to a balanced recursion tree.
Worst Case
The worst-case scenario occurs when the pivot selection results in highly unbalanced partitions, such as when the smallest or largest element is always chosen as the pivot. In this case, the time complexity degrades to O(n^2).
Optimizations
Several optimizations can be applied to improve the performance of Quicksort in practice.
Randomized Pivot Selection
Randomized pivot selection involves choosing a pivot randomly from the array. This reduces the likelihood of encountering the worst-case scenario and ensures that the average-case time complexity is more consistently achieved.
Median-of-Three Pivot Selection
The median-of-three method selects the pivot as the median of the first, middle, and last elements of the array. This approach often results in better partitioning and improves performance on average.
Tail Recursion Elimination
Tail recursion elimination can be applied to Quicksort to reduce the recursion depth, which is particularly useful for large arrays. This involves converting the tail-recursive calls into iterative loops.
Variants
Several variants of Quicksort have been developed to address specific use cases and improve performance.
Dual-Pivot Quicksort
Dual-pivot Quicksort, introduced by Vladimir Yaroslavskiy, uses two pivots instead of one. This variant can be more efficient than the traditional single-pivot Quicksort, especially for large arrays.
Three-Way Quicksort
Three-way Quicksort, also known as Dutch National Flag Quicksort, partitions the array into three parts: elements less than the pivot, elements equal to the pivot, and elements greater than the pivot. This variant is particularly effective for arrays with many duplicate elements.
Applications
Quicksort is used in various applications due to its efficiency and simplicity. It is commonly employed in:
- Database management systems for sorting records.
- Search algorithms for preprocessing data to enable faster searches.
- Computer graphics for tasks such as depth sorting in rendering pipelines.
See Also
References
- Hoare, C. A. R. (1961). "Quicksort". The Computer Journal. 5 (1): 10–16.
- Sedgewick, Robert (1978). "Implementing Quicksort Programs". Communications of the ACM. 21 (10): 847–857.