Selection in Programming: Difference between revisions
(Created page with "== Introduction == Selection in programming, also known as conditional statements or decision-making, is a fundamental concept that allows a program to choose different paths of execution based on certain conditions. This concept is essential in creating dynamic and responsive software applications. Selection constructs enable programs to make decisions, execute different code blocks, and handle various scenarios effectively. == Types of Selection Constructs == Select...") |
No edit summary |
||
Line 100: | Line 100: | ||
Pattern matching is particularly useful for deconstructing complex data structures and handling various cases elegantly. | Pattern matching is particularly useful for deconstructing complex data structures and handling various cases elegantly. | ||
[[Image:Detail-97539.jpg|thumb|center|A programmer writing code on a computer screen.|class=only_on_mobile]] | |||
[[Image:Detail-97540.jpg|thumb|center|A programmer writing code on a computer screen.|class=only_on_desktop]] | |||
== Best Practices for Using Selection Constructs == | == Best Practices for Using Selection Constructs == |
Latest revision as of 07:14, 8 August 2024
Introduction
Selection in programming, also known as conditional statements or decision-making, is a fundamental concept that allows a program to choose different paths of execution based on certain conditions. This concept is essential in creating dynamic and responsive software applications. Selection constructs enable programs to make decisions, execute different code blocks, and handle various scenarios effectively.
Types of Selection Constructs
Selection constructs can be broadly categorized into three types:
If Statements
The if statement is the most basic form of selection. It evaluates a condition and executes a block of code if the condition is true.
```python if condition:
# code to execute if condition is true
```
In many programming languages, the if statement can be extended with else and elif (else if) clauses to handle multiple conditions.
```python if condition1:
# code to execute if condition1 is true
elif condition2:
# code to execute if condition2 is true
else:
# code to execute if none of the conditions are true
```
Switch Statements
Switch statements, also known as case statements, provide a way to execute one block of code out of many based on the value of a variable. This construct is available in languages like C, C++, Java, and JavaScript.
```java switch (variable) {
case value1: // code to execute if variable equals value1 break; case value2: // code to execute if variable equals value2 break; default: // code to execute if variable does not match any case
} ```
Switch statements are particularly useful when dealing with multiple discrete values of a variable.
Conditional (Ternary) Operator
The conditional operator is a shorthand for simple if-else statements. It is available in languages like C, C++, Java, and JavaScript.
```javascript condition ? expressionIfTrue : expressionIfFalse; ```
This operator is useful for concise conditional assignments.
Advanced Selection Techniques
Nested Selection
Nested selection involves placing one selection construct inside another. This technique allows for more complex decision-making processes.
```python if condition1:
if condition2: # code to execute if both condition1 and condition2 are true else: # code to execute if condition1 is true and condition2 is false
else:
# code to execute if condition1 is false
```
Nested selection is commonly used in scenarios where multiple layers of conditions need to be evaluated.
Short-Circuit Evaluation
Short-circuit evaluation is an optimization technique used in logical expressions. In languages like C, C++, and Java, logical operators (&& and ||) evaluate the second operand only if necessary.
```java if (condition1 && condition2) {
// code to execute if both condition1 and condition2 are true
} ```
In this example, condition2 is evaluated only if condition1 is true. This technique can improve performance and prevent unnecessary computations.
Pattern Matching
Pattern matching is a powerful feature available in languages like Haskell, Scala, and Rust. It allows for more expressive and concise code by matching values against patterns.
```rust match value {
pattern1 => // code to execute if value matches pattern1, pattern2 => // code to execute if value matches pattern2, _ => // code to execute if value does not match any pattern
} ```
Pattern matching is particularly useful for deconstructing complex data structures and handling various cases elegantly.
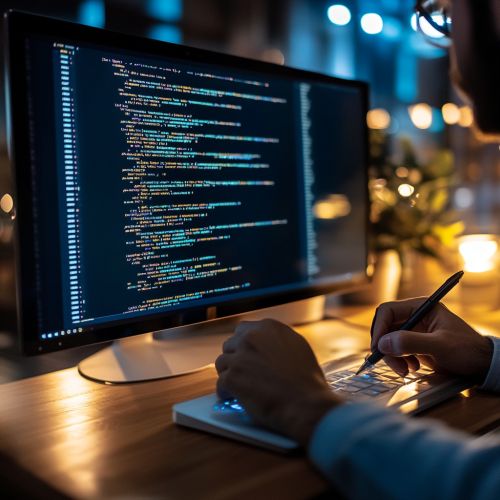
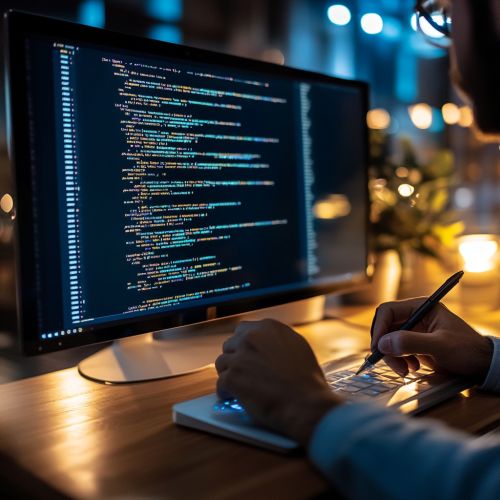
Best Practices for Using Selection Constructs
Readability
Maintaining readability is crucial when using selection constructs. Clear and concise code helps prevent errors and makes maintenance easier. Use meaningful variable names and avoid deeply nested conditions whenever possible.
Avoiding Redundancy
Avoid redundant conditions and code blocks. Consolidate conditions that lead to the same outcome to simplify the code.
```python if condition1 or condition2:
# code to execute if either condition1 or condition2 is true
```
Handling Edge Cases
Always consider edge cases and ensure that all possible scenarios are handled. This practice helps prevent unexpected behavior and improves the robustness of the program.
Using Switch Statements Appropriately
Switch statements are ideal for handling multiple discrete values of a variable. However, they should be used judiciously, as overly complex switch statements can become difficult to manage.
Leveraging Short-Circuit Evaluation
Use short-circuit evaluation to optimize performance and prevent unnecessary computations. This technique is especially useful in conditions involving expensive operations or function calls.
Common Pitfalls and How to Avoid Them
Off-by-One Errors
Off-by-one errors occur when the boundary conditions of loops or selections are incorrectly specified. These errors are common in programming and can lead to unexpected behavior.
```python for i in range(1, 10): # Correct range
# code to execute
```
Ensure that loop and selection boundaries are correctly defined to avoid off-by-one errors.
Unreachable Code
Unreachable code is code that can never be executed due to the structure of the selection constructs. This issue often arises from incorrect logic or redundant conditions.
```java if (condition) {
// code to execute
} else if (condition) {
// unreachable code
} ```
Review the logic of selection constructs to ensure that all code paths are reachable.
Logical Errors
Logical errors occur when the conditions in selection constructs do not accurately represent the intended logic. These errors can be challenging to detect and debug.
```python if condition1 and condition2:
# intended code
```
Carefully review and test conditions to ensure that they align with the intended logic.
Selection in Different Programming Paradigms
Procedural Programming
In procedural programming, selection constructs are used to control the flow of execution within procedures or functions. Languages like C and Pascal heavily rely on if statements and switch statements.
Object-Oriented Programming
In object-oriented programming (OOP), selection constructs are often used within methods to control the behavior of objects. Languages like Java and C++ use selection constructs to implement polymorphism and handle different object states.
Functional Programming
Functional programming emphasizes immutability and pure functions. Selection constructs in functional languages like Haskell and Scala often involve pattern matching and higher-order functions.
```haskell case expression of
pattern1 -> result1 pattern2 -> result2
```
Pattern matching in functional programming provides a concise and expressive way to handle different cases.
Logic Programming
In logic programming, selection constructs are used to define rules and relationships. Languages like Prolog use logical predicates to represent conditions and control the flow of execution.
```prolog condition1 :- condition2, condition3. ```
Selection constructs in logic programming are based on logical inference and backtracking.
Conclusion
Selection in programming is a fundamental concept that enables dynamic and responsive software applications. By understanding and effectively using selection constructs, programmers can create robust and efficient code. This article has explored various types of selection constructs, advanced techniques, best practices, common pitfalls, and their application in different programming paradigms.